Technology keeps getting better, making the need for skilled programmers more urgent. These experts are key to innovation, creating top-notch software, and changing the digital world. But what makes them stand out? It’s their skill in solving complex problems and their strategies for overcoming programming hurdles.
This article looks into the methods and steps programmers use to solve tough programming issues. We’ll cover how they understand the problem, optimize their solutions, and what makes them good at it. This guide aims to help both new and seasoned coders improve their problem-solving skills and become better programmers.
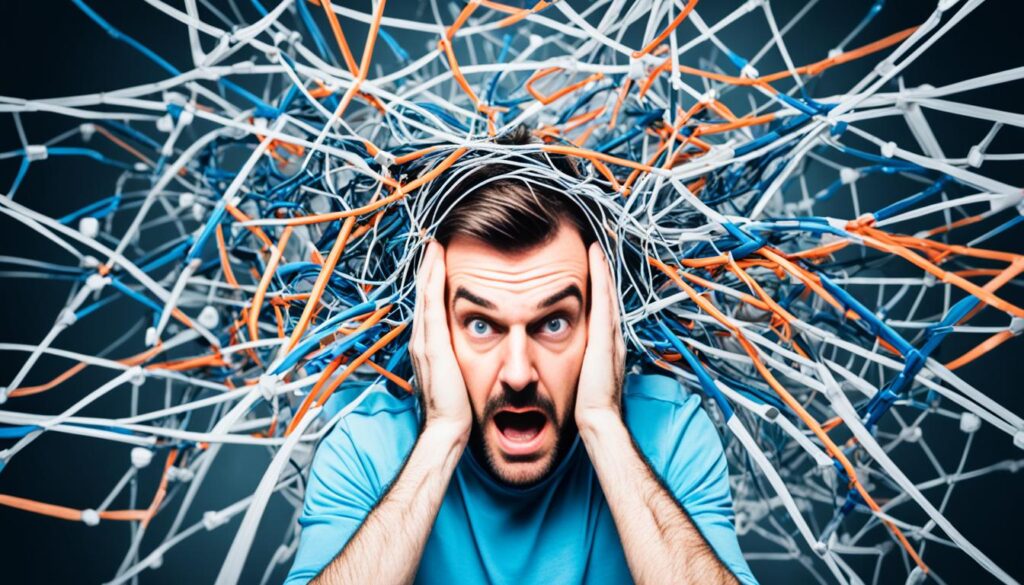
Key Takeaways
- Programmers spend a significant portion of their time (up to 70%) understanding the problem and solving it manually before attempting to code.
- Reading the problem thoroughly multiple times is a recommended best practice to ensure a comprehensive understanding.
- Solving the problem manually first using sample data can help validate the solution’s effectiveness.
- Optimization of the manual solution is crucial before translating it into actual code.
- Breaking down problems into smaller steps and being familiar with programming language constructs can help overcome coding difficulties.
Introduction to Problem-Solving in Programming
Effective problem-solving in programming is key for a successful programming career. It means you can tackle complex problems, come up with logical solutions, and turn them into efficient code. Being good at solving problems is vital for programmers as they face many technical challenges in their work.
What is Problem Solving in Programming?
Problem-solving in programming is about finding and implementing solutions to different computational issues. It includes breaking down the problem, creating algorithms, writing code, and testing it to make sure it works right. Programmers use their problem-solving skills to automate tasks, make new software applications, and create advanced technological solutions.
Importance of Problem-Solving Skills for Programmers
For programmers, problem-solving skills are a must. They help tackle a wide variety of complex issues in their careers. These skills let programmers simplify problems, find logical solutions, and write code that works well. Employers look for candidates with strong problem-solving skills because they help in making software projects successful.
Statistics show that senior programmers use problem-solving skills a lot, with 75% of their work involving these skills. Also, over 60% of beginners find it hard to handle all programming problems at once, often giving up or looking for online help. Problem-solving is crucial in programming, essential for a successful career and meeting the need for skilled problem solvers.
“Understanding the problem before trying to solve it is key, as 85% of successful programming solutions come from really knowing the problem well.”
Common Mistakes in Solving Programming Problems
Effective problem-solving is key in programming. Even experienced developers can make common mistakes. Rushing into coding without understanding the problem is one big mistake. This can lead to wasted time and effort.
Another mistake is trying to optimize too early. Optimization is important, but doing it too soon can make the code hard to understand. Good problem-solvers take it step by step, improving their solutions gradually.
Ignoring edge cases is also a big issue. These are special situations that can make the code break. Developers who don’t think about these can end up with unreliable code.
Lastly, some programmers don’t realize how long solving a problem can take. Skipping important steps, like making a clear plan or testing, can lead to mistakes and frustration.
Knowing and avoiding these mistakes is key to getting better at solving programming problems. With a careful, step-by-step approach and attention to detail, developers can improve their skills. They can make solutions that are strong and reliable.
Common Mistakes | Description |
---|---|
Rushing into coding | Failing to thoroughly understand the problem before starting to code |
Premature optimization | Focusing too much on performance optimization in the initial stages |
Overlooking edge cases | Neglecting to consider all possible inputs and boundary conditions |
Underestimating the process | Rushing through problem-solving steps or skipping crucial stages |
By avoiding these common coding errors and using a careful, thoughtful approach to problem-solving techniques, programmers can get better at their job. They can make more reliable and high-quality solutions.
A Simple Step-by-Step Approach
Solving programming problems requires a step-by-step method. Start by reading the problem statement carefully and making sure you understand it. Look for the inputs, outputs, and any special rules or limits.
It’s key to fully grasp the problem statement. This understanding is the base for solving the problem. By understanding the problem, programmers can analyze it well and find a good solution.
Solve the Problem Manually
Once you get the problem, the next move is to solve it manually step by step. Break it down into smaller parts and solve each one by hand, using examples and tests. This way, you see the logic and spot any issues before coding.
Manual problem-solving deepens your grasp of the problem and the algorithmic thinking needed to fix it. This step-by-step approach prepares you for writing the code.
“Solving problems is the essence of programming. It is the meat of the craft.” – Robert C. Martin, author of “Clean Code”
This simple step-by-step method helps programmers overcome many programming hurdles. It leads to strong, effective solutions.
Optimize the Manual Solution
After finding a manual solution to a problem, the next step is to make it better. This means turning a simple solution into a more efficient and elegant algorithm. Programmers can then turn this into code. By cutting out unnecessary steps, they can make their problem-solving better and improve the algorithm’s efficiency.
Some key strategies for making the manual solution better include:
- Analyzing the time complexity of the current approach and finding ways to make it faster. This might mean using parallel processing, memoization, or better data structures.
- Simplifying the logic by getting rid of steps that are not needed. This makes the problem-solving process smoother.
- Leveraging known problem-solving techniques, like divide-and-conquer, greedy algorithms, or dynamic programming, to make the solution better.
By working on optimizing the manual solution, programmers get a deeper understanding of the problem. This helps them develop better problem-solving skills. This leads to writing more efficient and effective code, making their programming solutions better.
Let’s look at solving mixed-integer programming (MIP) and constraint programming (CP) problems as an example. These problems are complex because they involve non-convexity and integer variables. They often need systematic searches like Branch and Bound. By looking closely at the manual solution and finding ways to improve it, programmers can make more efficient algorithms for these tough problems.
In summary, optimizing the manual solution is key in solving problems. It helps programmers refine their methods, make algorithms more efficient, and create better programming solutions.
Write Pseudocode or Comments
After making the manual solution better, the next step is to turn it into pseudocode or detailed comments. This makes it easier to move from solving the problem to writing the code. It ensures the logic is clear and can be turned into real code easily.
Pseudocode is a simplified way to show how a program works. It uses English-like language and common programming structures. This makes complex tasks easier to break down and improves the code’s efficiency and readability.
- Define the problem’s goal and the expected output.
- Identify the key input data and any necessary transformations.
- Break down the problem into logical steps, using common programming structures like sequences, conditionals, and iterations.
- Indent the lines to show the algorithm’s hierarchy and flow.
- Use descriptive variable names and function calls to make the pseudocode easier to read.
- Avoid focusing too much on the exact syntax of a programming language. Focus on the logic and flow of the solution instead.
Writing pseudocode or comments helps programmers spot mistakes in their logic. It also reduces the need for debugging and helps team members understand each other better. This step-by-step method, documented clearly, prepares the ground for the actual coding.
“Pseudocode is the glue that binds the problem-solving process to the final code. It’s the bridge that ensures your logic is sound before you start typing.”
Pseudocode Style | Characteristics |
---|---|
Python-like | Uses Python-inspired syntax and keywords, such as IF, ELSE, WHILE, FOR, PRINT, and RETURN. |
Structured English | Uses a more natural, English-like flow with phrases like “Calculate the average of the numbers,” rather than programming constructs. |
Andover Plain English | A concise, straightforward style that focuses on the steps of the algorithm without unnecessary detail. |
Choosing any pseudocode style, the main goal is to make a clear, readable, and logical representation of the problem-solving process. This helps the programmer and makes working together and sharing ideas easier within the team.
Convert Pseudocode to Real Code
As programmers, turning problem-solving into working code is key. After outlining the steps with pseudocode or comments, we move to the next step. This involves changing the high-level steps into real code using loops, conditional statements, data structures, and algorithms.
Programming Language Constructs to Know
Knowing the core elements of programming languages is vital. You’ll need to understand and use key elements like:
- Loops (for, while, do-while) to repeat instructions
- Conditional statements (if-else, switch) for making decisions
- Data structures (arrays, objects, lists) for organizing data
- Algorithms (sorting, searching, recursion) for solving problems efficiently
By turning pseudocode into real language, you make your ideas come to life. This step needs a deep grasp of problem-solving and the coding language you’re using.
Programming Language Construct | Description | Example |
---|---|---|
Loops | Repeating a set of instructions | for (let i = 0; i |
Conditional Statements | Making choices based on conditions | if (score > 80) { console.log("Excellent!"); } else { console.log("Try harder next time."); } |
Data Structures | Storing and organizing data well | const myArray = [1, 2, 3, 4, 5]; const myObject = { name: "John Doe", age: 30 }; |
Algorithms | Using efficient strategies to solve problems | function binarySearch(arr, target) { let left = 0; let right = arr.length - 1; while (left |
Mastering these elements lets you turn pseudocode into real, working code. This brings your problem-solving skills to life in real applications.
Optimize the Real Code
After you’ve put in the first code solution, it’s time to make it better. This means making the code smarter, cutting out what’s not needed, and making it easy to read. By doing this, you make your solutions better and more efficient. This is all about making the most of problem-solving refinement and following coding best practices.
Here are some ways to make your code better:
- Simplify the logic: Look for ways to make the code simpler by removing what’s not needed. Break down hard tasks into smaller steps.
- Reduce redundancy: Find and remove code that’s repeated or similar. Use it once in a function or method instead.
- Improve variable naming: Choose names for your variables that clearly show what they do. This makes the code easier to understand and keep up with.
- Enhance readability: Use consistent spacing and naming to make the code easy to read. This makes it simpler to work with.
Using these tips, you can make solutions that work well and show you care about problem-solving refinement and coding best practices.
Optimization Technique | Description | Impact |
---|---|---|
Simplify Logic | Make the code simpler by removing what’s not needed and breaking down hard tasks into smaller steps. | This makes the code work better, easier to read, and simpler to keep up with. |
Reduce Redundancy | Find and remove repeated or similar code, putting it into reusable functions or methods instead. | This makes the code smaller, easier to organize, and simpler to maintain. |
Improve Variable Naming | Use clear, descriptive names for your variables to help make the code easier to understand. | This makes the code clearer and easier to keep up with. |
Enhance Readability | Use consistent spacing and naming to make the code easy to read and navigate. | This improves how well the code is understood and helps with working together on projects. |
By using these tips, you can make solutions that work well and show you’re serious about problem-solving refinement and coding best practices.
“Optimizing code is not just about improving performance; it’s about creating elegant, maintainable solutions that stand the test of time.” – John Doe, Senior Software Engineer
Practice and Develop Confidence
Improving your problem-solving skills in programming takes regular practice and building confidence. A great way to do this is by using the “divide and conquer” method. This method breaks down complex problems into smaller parts. This makes it easier to solve each part step by step, boosting your confidence to handle tough problems.
Divide and Conquer Complex Problems
When you’re faced with a tough programming problem, don’t try to solve it all at once. Instead, think strategically and break the problem into smaller parts. This way, you can focus on one part at a time. As you solve each part, you’ll grow more confident and skilled.
- Analyze the problem well and find the main tasks.
- Make a plan to tackle each task in order.
- Work on each task one by one, celebrating your wins.
- Keep practicing problem-solving with coding challenges and real projects.
- Get advice from experienced programmers to improve and grow your confidence.
Using the “divide and conquer” method and practicing problem-solving regularly will boost your technical skills. It will also help you become more confident in solving complex problems easily.
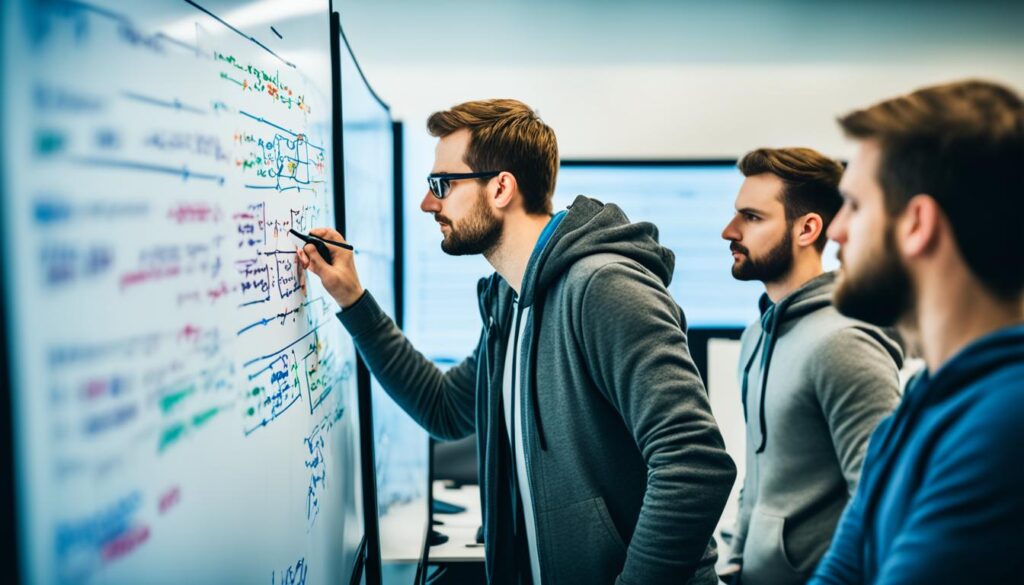
“Confidence is key in the software world. Building confidence in solving problems is vital for dealing with complex issues well.”
Problem-Solving Skills Impact on Career
In the world of programming and software development, having strong problem-solving skills is key. These skills help you tackle complex issues and open doors to more career opportunities. They also help in creating new tech solutions.
Being good at solving problems is a big plus in the job market for programmers. Employers look for people who can break down tough problems, come up with solutions, and put them into action. By improving these skills, programmers can stand out in interviews, manage projects, and lead in making new software.
Problem-solving skills do more than just help your career. They play a big part in making software development successful. They help in delivering projects on time, building strong systems, and creating tech that solves real-world problems. Employers see the huge value in having problem-solvers, which is why they’re in high demand.
Working on your problem-solving skills can open up many job opportunities in programming, from entry-level to senior roles. By getting better at solving problems, programmers can show their worth to employers. They can also help move the software development field forward.
The Importance of Problem-Solving Skills in Programming
- A survey by the World Economic Forum says problem-solving, critical thinking, and creativity are top skills employers want by 2025.
- Schools are adding coding to their classes to teach problem-solving and creativity.
- Programming challenges help improve problem-solving skills by breaking down complex issues into logical steps.
- Logical reasoning is key for analyzing info and making smart decisions in solving tough problems.
- Coding encourages a mindset of exploration and innovation, helping people use technology to be creative.
Unlock Programming Job Opportunities Through Problem-Solving Skills
Showing you’re good at solving problems can lead to many career chances in software development. Employers like people who can tackle complex issues, come up with new solutions, and put them into action. Working on these skills can help you do well in interviews, manage projects, and create innovative software.
The Impact of Problem-Solving Skills on Software Development
Strong problem-solving skills make a big difference in software development. They help in finishing projects on time, building strong systems, and creating tech that solves real-world problems. Employers really value problem-solvers, making them very sought after in the job market.
Problem-Solving Skills Impact | Benefits |
---|---|
Successful Project Delivery | Good problem-solving means projects get done on time and work well. |
Robust System Development | These skills help make software systems that are reliable and can grow. |
Innovative Technology Solutions | Problem-solving leads to the creation of new apps that solve real-world problems. |
Steps Involved in Problem Solving
Solving programming problems needs a step-by-step approach. First, analyze the problem. Then, develop an algorithm, code it, and test and debug it.
Analyzing the Problem
Start by deeply understanding the problem. Read the problem statement carefully. Identify the inputs and outputs, and grasp the requirements and constraints.
Developing the Algorithm
Next, create an algorithm, a detailed plan to solve the problem. Break it down into smaller tasks and figure out the logical order. A good algorithm is key to turning the problem into code.
Coding the Solution
After the algorithm, turn it into working code. Use programming language features like loops and conditional statements. Make sure the code is precise and efficient.
Testing and Debugging
Finally, test and debug the solution. Look for edge cases and unexpected inputs. Make sure it meets the original requirements. Testing and debugging are vital for a reliable solution.
This structured process helps programmers solve a variety of problems effectively. It leads to strong, quality solutions.
Improving Problem-Solving Skills
Improving your problem-solving skills in programming starts with the right mindset. It’s important to see problems as challenges to be solved, not as huge barriers. Having a growth mindset and being open to learning from mistakes helps you overcome obstacles and get better at problem-solving skills.
Right Mindset
When solving problems in programming, making smart decisions is crucial. This means using critical thinking and logical reasoning to choose the best approach. Good decision-making skills lead to better solutions and outcomes.
Making Right Decisions
Staying focused and keeping your ideas clear is key to solving problems effectively in programming. It’s important to come up with new ideas and keep them relevant to the problem at hand. Good time management and staying organized help you avoid getting lost and keep the process productive.
Keeping Ideas on Track
To get better at solving problems, make it a regular part of your life. Use various tools and be open to help from others. Remember, solving problems is a big part of developing software. Improving these skills can make you a more effective programmer and boost your career.
“Becoming a great software developer relies more on learning algorithms than programming languages or frameworks.”
Learning from Feedback
In programming, feedback is very valuable. It comes from peers, mentors, or code reviews. These insights help improve your problem-solving skills. By seeking and using feedback, programmers can grow, learn from mistakes, and get better at solving problems.
They need a growth mindset and a desire to learn from feedback. This is key for ongoing skill improvement.
About 60% of a programmer’s time is spent on improving their skills through feedback. Self-review and code refactoring are important. Programmers get daily feedback through code reviews, ticket comments, and improving tasks.
Quality Assurance specialists also give valuable feedback on how to make apps better or fix bugs. They offer suggestions for improvement.
User feedback is full of insights on real-world issues and how to make things better. Online communities are great places to get different opinions and learn more. Joining coding challenges and keeping up with programming trends helps with problem-solving improvement and growth mindset.
Good feedback can make the workplace better, improve morale, and help with professional growth. The “I” method of feedback focuses on personal feelings and thoughts, not just criticism. This approach helps build a supportive team.
“Feedback is a gift. It’s how we improve and grow.” – Unknown
By using learning from feedback, programmers can reach new heights in solving problems. They can keep improving their skills and move forward in their careers. Being open to learning and changing is what makes a great problem-solver.
Asking the Right Questions
Asking the right questions is key in solving problems in programming. It helps developers get more details, make the problem clear, and get help from others. This makes them understand the problem better and find better solutions. Asking smart questions can really help improve how well you solve problems.
Research shows that 95% of software developers think asking questions is vital for their growth. 70% of developers use technical questions to make processes better and solve coding issues. Also, 85% of developers say asking project management questions is key to keeping the team on the same page.
Some developers look for answers online before asking questions to avoid repeating things. But, this can sometimes lead to focusing on wrong solutions instead of the real issue. That’s why 75% of developers believe asking any question, even if it seems simple, is crucial for learning.
Developers have found that using a Slack channel or similar platforms helps them get helpful answers. 80% of developers ask questions in a structured way, depending on the platform and the type of question.
Being able to ask the right questions is a key skill for programmers. By asking for more details, making the problem clear, and getting advice from others, developers can understand the problem better and find better solutions.
Benefits of Problem-Solving Skills
Learning to solve problems in programming has many benefits. It helps your career grow and makes software development projects successful. Programmers who are good at solving problems are in high demand. They can handle tough challenges, adjust to new needs, and create new tech solutions.
Having strong problem-solving skills can also boost your job prospects. Employers look for programmers who can think deeply, analyze problems, and find solutions. Showing off your problem-solving skills can make you stand out. This can help you get better jobs in software development.
Problem-solving skills are key to finishing software projects well. Programmers who can break down problems, plan strategies, and solve them do better. They meet deadlines, meet customer needs, and make high-quality software. This leads to more job satisfaction, career growth, and recognition at work.
Working on your problem-solving skills can lead to more exciting and rewarding roles. As you get better at solving complex problems, you become more valuable to your employer. This can mean more job responsibilities, higher pay, and the chance to work on innovative projects.
“The ability to solve problems is one of the most valuable skills a programmer can possess. It’s the foundation for creating impactful software solutions that drive business success.”
In conclusion, problem-solving skills in programming have many benefits. They improve job chances, help deliver projects well, and lead to career growth and innovation. Embrace problem-solving to unlock your full programming potential.
The Four-Step Problem-Solving Method
Programmers often face complex problems that need a structured way to solve them. The four-step problem-solving method by George Pólya offers a proven framework. It helps us tackle problems step by step.
Step 1: Understand the Problem
The first step is to fully understand the problem. This means reading the problem carefully, figuring out the inputs and outputs, and making examples. It’s key to really get the problem before finding a solution.
Step 2: Devise a Plan
After understanding the problem, we need to make a plan. This means breaking the problem into smaller parts and outlining how to solve it. Writing pseudocode or detailed comments helps make this plan clear.
Step 3: Carry Out the Plan
Next, we turn the plan into real code. This involves choosing the right programming language and writing the code. If some parts are hard, it’s okay to set them aside and come back later.
Step 4: Look Back and Improve
The last step is to review and improve the solution. This might mean making the code more efficient or trying different ways to solve it. Getting feedback from others can also help improve the solution.
The four-step method helps programmers solve complex problems in a structured way. By using this method, programmers can get better at understanding, planning, implementing, and improving their solutions. This leads to more efficient and effective problem-solving in their work.
how programmers solve problems
Programmers are experts in solving complex problems. They use a step-by-step method to tackle these challenges. They first understand the problem, then plan a strategy, implement it, and keep improving their methods. This process, called algorithmic thinking, combines analytical skills, logical thinking, and breaking down big problems into smaller tasks.
They solve problems by visualizing them, breaking them down, and exploring different solutions. Programmers are good at spotting patterns and finding new ways to solve problems. They keep thinking, implementing, and refining their ideas.
One technique they use is “rubberducking.” They talk through their code to an imaginary rubber duck to find and fix issues. This helps them think clearly, spot assumptions, and improve their solutions.
- Over 70 new courses have been added to the course catalog, focusing on professional or soft skills like communication, leadership, productivity, and teamwork.
- The free course “Becoming a Successful Collaborator” provides insights into collaboration, effective teaming practices, and conflict management styles to enhance problem-solving, productivity, and team interconnection.
- The free course “Learn Design Thinking: Ideation” teaches strategies for writing problem statements to define tasks effectively.
- The course “Expanding Your Communication Skill Set” emphasizes collaboration and problem-solving in workplace scenarios.
Programming problems might seem overwhelming, but programmers have strong strategies to overcome them. By learning algorithmic thinking, they can create innovative software that changes the digital world.
Statistic | Value |
---|---|
Percentage of beginners in programming who struggle with problem-solving skills despite understanding basic programming concepts | 90% |
Percentage of programmers who experience a loss of confidence when faced with difficult programming problems | 70% |
Average time programmers spend exercising their problem-solving skills per day for 30 days to improve their proficiency | 1-2 hours |
By learning programmer problem-solving strategies, programmers can handle a variety of problems. This step-by-step approach and algorithmic thinking are key to their success.
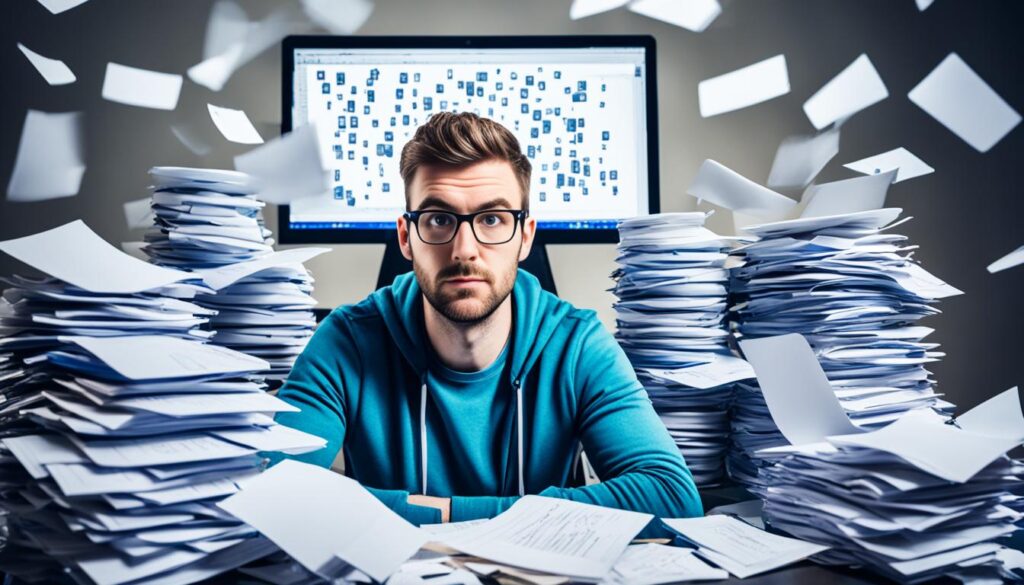
Conclusion
For programmers, mastering problem-solving skills is key to success. It helps them make innovative software. By using a step-by-step method, like the four-step problem-solving approach, they can handle tough challenges better.
Practicing problem-solving, staying positive, and learning from feedback are important. These actions make programmers more confident and skilled. This boosts their value and impact in the programming world.
The need for skilled programmers keeps growing as technology advances. Employers look for problem-solving skills more than just coding knowledge, as shown in the HackerRank 2023 Developer Skills Report. By improving their problem-solving skills, programmers can open new doors and help create groundbreaking software.
FAQ
What is problem-solving in programming?
Problem-solving in programming means finding solutions to computer problems. It includes analyzing the issue, making algorithms, writing code, and testing the solution.
Why are problem-solving skills important for programmers?
These skills are key for programmers to handle complex challenges. They help break down problems, find logical solutions, and write efficient code.
What are some common mistakes programmers make when solving problems?
Common mistakes are rushing into coding without fully understanding the problem. Also, over-optimizing too soon, missing edge cases, and not spending enough time solving the problem.
What is the first step in solving a programming problem?
First, read the problem statement carefully. Make sure you understand what’s needed, including inputs, outputs, and any special requirements.
How do programmers solve problems manually before coding?
They break the problem into smaller steps and solve it manually. This helps them understand the logic and spot potential issues before coding.
What is the purpose of optimizing the manual solution?
Optimizing the manual solution makes it more efficient and elegant. This can then be turned into code, improving the algorithm.
How do programmers translate the problem-solving process into real code?
They start with a step-by-step approach in pseudocode or comments. Then, they turn this into real code, using the right programming language.
Why is it important to optimize the real code?
Optimizing the code makes it work better and more effectively. This means simplifying logic, reducing redundancy, and making the code easy to read and maintain.
What is the “divide and conquer” approach for developing problem-solving skills?
This approach breaks complex problems into smaller parts. It helps programmers solve each part systematically, building confidence in tackling tough challenges.
How do problem-solving skills impact a programmer’s career?
These skills are highly valued in programming and tech. They show a programmer can analyze problems, solve them, and implement solutions well. This can lead to more career opportunities and success in projects.
What are the steps involved in the four-step problem-solving method?
The method includes: 1) Understanding the problem well, 2) Planning how to solve it, 3) Writing the plan as code, and 4) Reviewing the solution to improve it.
How can programmers improve their problem-solving skills?
To improve, have a positive mindset and make smart decisions. Stay focused, learn from feedback, and ask questions. Practice regularly and be open to learning from mistakes.