As a programmer, knowing about memory is key. It helps you make your code better, use resources wisely, and create fast applications. This guide will cover the basics of memory management. You’ll learn about memory allocation, virtual memory, cache memory, and how to make your memory use better. By the end, you’ll know what every programmer should understand about memory.
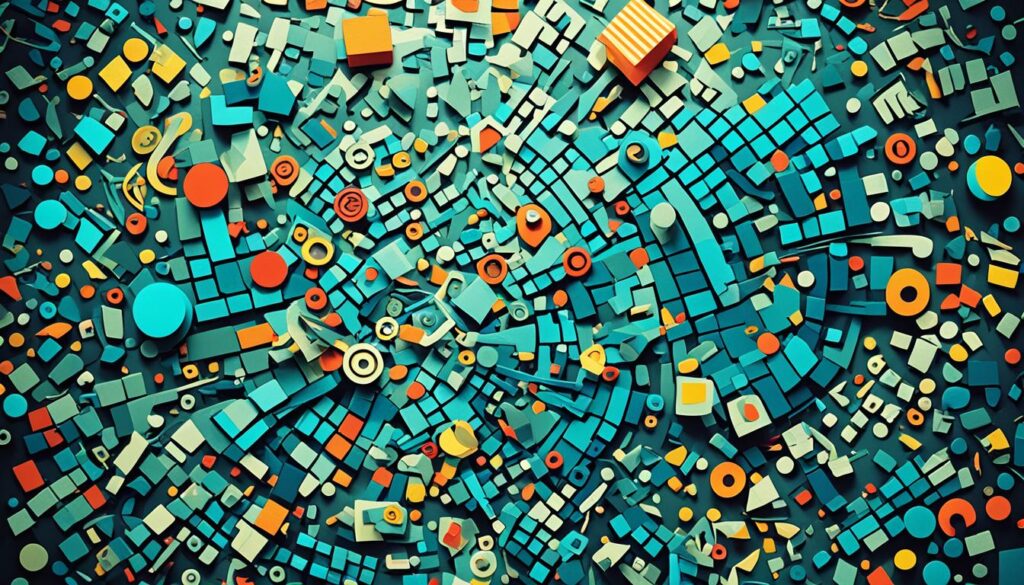
Key Takeaways
- Understand the basics of RAM, ROM, and the differences between volatile and non-volatile memory.
- Explore the concepts of static and dynamic memory allocation, heap and stack memory, and their importance in programming.
- Dive into virtual memory concepts, including paging and segmentation, and how they affect application performance.
- Learn about cache memory and how to use it better.
- Get to know memory profiling and debugging tools to find and stop memory leaks.
- Learn best practices and common mistakes in memory management across different programming languages.
- Keep up with new trends in memory technologies and how they might change software development.
Introduction to Memory Basics
To understand computer programming well, knowing about memory is key. At the heart of every computer, there’s a mix of different memories, each with its own role. We’ll look into the basics of memory and see how RAM and ROM differ. We’ll also talk about the difference between volatile and non-volatile memory.
Understanding RAM and ROM
Random Access Memory (RAM) is the main memory of a computer. It’s volatile, meaning it forgets its data when turned off. RAM lets programs run fast by accessing data quickly. Read-Only Memory (ROM), on the other hand, keeps its data even when off. It stores the computer’s basic startup info and is crucial for starting up.
Volatile vs. Non-Volatile Memory
Volatile and non-volatile memory differ in how they keep data. Volatile memory, like RAM, needs power to keep its data. If the power goes out, the data is lost. Non-volatile memory, such as ROM and flash memory, keeps data even without power. This makes it perfect for storing important system info and data that needs to last.
Knowing about these memory types is vital for programmers. It helps them write better code and manage data well. By understanding volatile and non-volatile memory, programmers can make smart choices about how to store and manage data.
Next, we’ll dive deeper into memory management. We’ll look at static and dynamic memory allocation and how they affect program performance and stability.
Memory Management
Managing memory well is key in programming. It helps programs use system resources wisely and avoid problems like memory leaks. There are two main ways to manage memory: static and dynamic allocation.
Static Memory Allocation
Static memory allocation sets aside a fixed amount of memory for a program at compile time or startup. This method is easy to understand and predictable. It’s great for global variables and data that doesn’t change size while the program runs.
Dynamic Memory Allocation
Dynamic memory allocation lets a program ask for and give back memory as it goes. This is super useful for data that changes size, like arrays or linked lists. It uses functions like malloc()
, calloc()
, and free()
in languages like C and C++.
Choosing between static and dynamic memory allocation depends on the program’s needs and the data it handles. Static allocation is simpler and can be faster but might limit flexibility. Dynamic allocation is more flexible but needs careful handling to prevent memory leaks.
Characteristic | Static Memory Allocation | Dynamic Memory Allocation |
---|---|---|
Memory Allocation | Fixed at compile-time | Adjustable at runtime |
Memory Usage | Predictable and efficient | Flexible but requires more management |
Typical Use Cases | Global variables, constants, and other fixed-size data | Dynamic data structures, arrays, and other variable-size data |
Knowing about static and dynamic memory allocation helps programmers make smart choices. This ensures their apps use memory well and avoid common problems.
Heap and Stack Memory
In computer programming, knowing about heap and stack memory is key for developers. These areas are crucial for how programs store and use data. They affect how well and efficiently applications work.
The stack memory holds function calls, local variables, and parameters. It uses a Last-In-First-Out (LIFO) system. This means the newest item is the first to be taken out. It’s great for storing small, temporary data because it’s quick to access.
The heap memory is for bigger, complex data. It doesn’t follow a set order for storing and getting data back. Instead, it uses dynamic memory allocation. This means memory is given and taken back as the program runs. It’s perfect for objects, arrays, and other complex data that need flexible memory.
How the stack and heap memory work affects how programs run and perform. Memory allocation on the stack is quicker and simpler than on the heap. But, the stack can only hold so much data before it overflows, causing errors.
The heap can grow and change size, but it’s more complex. It can lead to memory issues like fragmentation and leaks. Developers must handle the heap memory well to avoid these problems.
Knowing the differences between stack and heap memory helps programmers make better memory choices. By using each memory type wisely, developers can make their software run better, last longer, and be more successful.
Virtual Memory Concepts
In computing, virtual memory is key to making computers work better with their memory. It acts as a bridge between programs and hardware. This layer helps manage memory well, letting programs use more memory than they physically have.
Paging and Segmentation
Paging and segmentation are the main ways virtual memory works. Paging breaks physical memory into blocks called pages. Segmentation splits virtual memory into segments of different sizes. Together, they make managing memory smooth and flexible.
Paging lets the operating system move pages between memory and disk storage. This is called the page file or swap file. If a program needs a page not in memory, the system can get it from the disk. This makes it seem like programs have more memory, boosting performance.
Segmentation offers a flexible way to manage memory. It divides virtual memory into segments that fit different program parts. This reduces memory waste and makes the system run better.
Concept | Description |
---|---|
Paging | Dividing physical memory into fixed-size blocks called pages, allowing for swapping between memory and disk storage. |
Segmentation | Partitioning virtual memory into variable-sized segments, providing a more flexible approach to memory management. |
Paging and segmentation together make virtual memory powerful. They let programs use more memory than they have physically. This improves how well the system works and runs efficiently.
Memory Leaks and Their Impact
In software development, managing memory well is key. Memory leaks are a big problem that can slow down or crash programs. They happen when a program doesn’t free up memory it doesn’t need anymore.
Identifying and Preventing Memory Leaks
A memory leak makes a program use more and more memory over time. This can cause slow performance, crashes, or even make the program fail completely.
Finding memory leaks is hard because they can be tricky to spot. Programmers use tools and methods to track them down. By knowing what causes leaks and how to stop them, developers can keep their code efficient with memory.
- Use dynamic memory allocation and deallocation properly to avoid memory leaks.
- Implement a consistent memory management strategy, such as a reference counting system or garbage collection, to automatically handle memory allocation and deallocation.
- Regularly review and test your code to identify and fix any memory management issues.
- Utilize memory profiling tools to identify and diagnose memory leaks in your application.
- Adopt coding best practices, such as properly encapsulating memory-related operations and avoiding global variables, to minimize the risk of memory leaks.
Causes of Memory Leaks | Strategies to Prevent Memory Leaks |
---|---|
Failure to properly deallocate memory Circular references in object-oriented programming Incorrect usage of memory management functions Forgetting to release resources (e.g., file handles, database connections) | Implement a memory management strategy (e.g., reference counting, garbage collection) Regularly review and test your code for memory management issues Use memory profiling tools to identify and diagnose memory leaks Follow coding best practices to minimize the risk of memory leaks |
Understanding memory leaks and how they affect programs helps programmers make their software run better. By knowing how to spot and stop leaks, they can make sure their software works well and gives a good experience to users.
“Effective memory management is the key to building robust and scalable software applications.”
Cache Memory and its Significance
In today’s computing world, cache memory is key to how fast apps run. It’s a special memory that links the processor to the main memory. This link makes accessing data and instructions much faster.
The memory hierarchy has different levels, with cache memory at a vital spot. Knowing how cache memory works and its role helps programmers make their code better. This leads to better cache performance.
The Levels of Cache Memory
Cache memory has several levels, each with its own job in the memory setup. The main cache levels are:
- Level 1 (L1) Cache: This is the smallest and fastest cache, right next to the processor. It gives super-fast access to data and instructions used a lot.
- Level 2 (L2) Cache: L2 is bigger and a bit slower than L1 but still much quicker than the main memory. It helps close the speed gap between the processor and main memory.
- Level 3 (L3) Cache: L3 is the biggest and slowest cache level but still much faster than main memory.
How these cache levels are placed and used in the memory hierarchy greatly affects a computer’s performance.
Leveraging Cache Memory for Performance Optimization
Developers can improve their apps’ cache performance with several strategies. These include:
- Using data structures and algorithms that use the cache well
- Managing memory to keep data close together
- Programming in ways that use the cache, like avoiding random memory access and using prefetching
By understanding cache memory and its place in the memory hierarchy, programmers can make their apps run better. This means they can give users a great experience.
Cache Level | Size | Access Time | Purpose |
---|---|---|---|
L1 Cache | 32-256 KB | 1-2 CPU cycles | Fastest access to frequently used data and instructions |
L2 Cache | 256 KB – 8 MB | 5-20 CPU cycles | Bridge the performance gap between the processor and main memory |
L3 Cache | 2 MB – 64 MB | 20-40 CPU cycles | Larger cache to handle the demand for data and instructions |
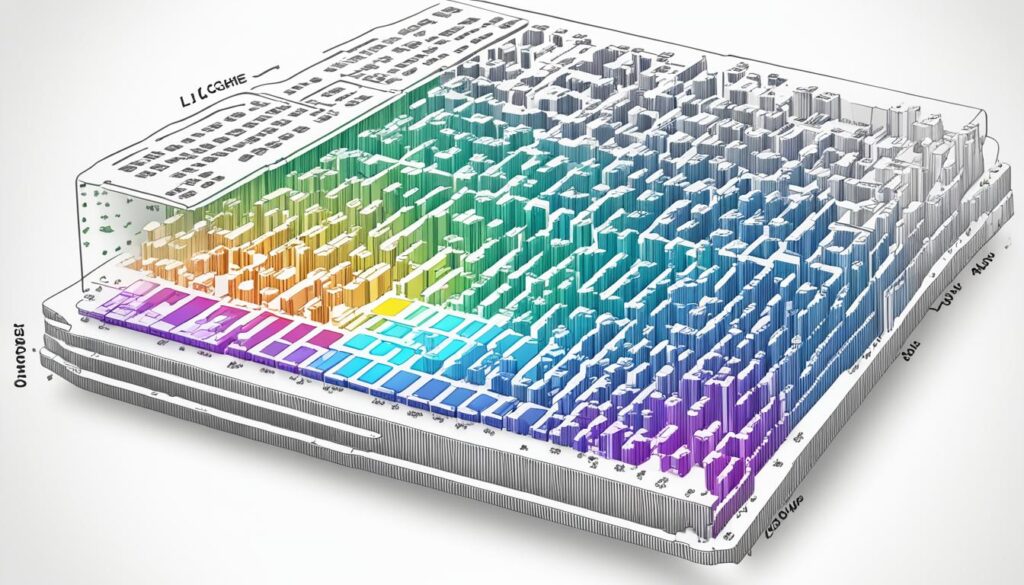
“Cache memory is the unsung hero of modern computing, providing a crucial performance boost that often goes unnoticed by end-users.”
Memory Optimization Techniques
In programming, using memory wisely is key to making apps run smoothly. It helps avoid crashes and makes users happy. This part will look at ways to use memory better, focusing on picking the right data structures and algorithms.
Data Structure and Algorithm Considerations
Choosing the right data structures and algorithms affects how much memory a program uses. Developers should pick data structures that fit their app’s needs. For instance, a memory-efficient data structure like a hash table can be better than an array for big datasets.
Algorithms also play a big role in how much memory a program uses. Using efficient algorithms can save a lot of memory. For example, in-place sorting algorithms or memoization can help. Developers should aim for algorithms that use memory wisely without slowing down the program.
Programmers can also use memory pooling. This means setting aside memory blocks and using them over and over. It helps reduce memory waste and makes managing memory better.
Using smart pointers and automatic memory management tools like garbage collection makes managing memory easier. It also lowers the chance of memory bugs.
By thinking about how data structures and algorithms use memory, and using smart memory management, developers can make apps that use memory well. This leads to better performance and fewer problems.
“Memory optimization is not just an optimization technique – it’s a fundamental aspect of creating high-performing, reliable software.”
Memory Profiling and Debugging
Finding and fixing memory problems can be tough, but programmers have many tools to help. Tools for memory profiling and debugging let developers check and improve how their apps use memory. This makes sure apps work well and reliably.
Memory profiling tools give deep insights into how much memory an app uses. They help spot memory leaks and high memory use. These tools track where memory is being used and show reports on trouble spots.
- Popular memory profiling tools include Microsoft Visual Studio Profiler, Valgrind, and Intel VTune Amplifier.
- These tools help programmers see how their code uses memory. They spot memory-heavy parts and guide them to optimize memory use.
Memory debugging tools also aid in fixing memory issues. They find and report on problems like null pointer errors and memory leaks.
- Memcheck, part of Valgrind, is a strong tool for finding memory errors.
- Microsoft Visual Studio’s debugger lets programmers check memory use step by step.
Using these tools, programmers can deeply understand their app’s memory use. They can fix performance issues and make sure their software works well and reliably.
“Effective memory management is a critical aspect of software development, and the right tools can make all the difference in identifying and resolving memory-related issues.”
As software challenges grow, knowing how to profile and debug memory will be key. By keeping up with new tools and best practices, developers can make their code better. This leads to apps that work great and give users a good experience.
What Programmers Should Know About Memory?
As a programmer, knowing how memory works is key to writing efficient code. It’s important to understand both the best ways to manage memory and the common mistakes to avoid. Let’s dive into what every programmer should know about memory.
Best Practices for Memory Management
Good memory management is crucial for reliable and fast software. Here are some important tips:
- Allocate and deallocate memory carefully: Dynamically allocate memory when needed, and ensure timely deallocation to prevent memory leaks.
- Optimize data structures and algorithms: Choose data structures and algorithms that use less memory and work well with memory access.
- Leverage caching effectively: Use cache memory to make data retrieval faster and reduce main memory access.
- Monitor memory usage: Regularly check and profile your application’s memory use to spot and fix any problems.
- Understand memory allocation models: Know the memory allocation models in your programming language, like stack and heap memory.
Common Memory Issues to Avoid
It’s also key to know about common memory problems that can affect your programs. Some of these include:
- Memory leaks: Not properly freeing memory can cause memory leaks, making your app use more and more memory over time.
- Buffer overflows: Writing past allocated memory can lead to buffer overflows, which might cause security issues or system crashes.
- Dangling pointers: Using memory locations that have been freed can cause dangling pointers, leading to unpredictable results.
- Fragmentation: Repeatedly allocating and freeing memory can cause memory fragmentation, making memory use less efficient.
Knowing these common memory issues and how to prevent them helps you write more solid and reliable software. This way, your apps will manage system memory better.
Mastering memory management is vital for any programmer. By following best practices and avoiding common mistakes, you can make apps that run smoothly, use memory well, and give users a great experience.
Memory Management in Different Programming Languages
As programmers, knowing how memory is managed in various programming languages is key. Each language has its own way of handling memory. This knowledge helps in picking the right tools for your projects.
Memory Management in C
In C, managing memory is done by the programmer. You use functions like malloc() and free() to allocate and free memory. This gives you control but requires careful handling to avoid memory leaks and errors.
Memory Management in Java
Java uses garbage collection for automatic memory management. The JVM takes care of memory allocation and deallocation for you. While this makes coding easier, it can lead to unpredictable garbage collection and performance issues.
Memory Management in Python
Python combines automatic and manual memory management. The reference counting system automatically handles memory, but you can use the ctypes module for more control. This mix gives you flexibility while still offering automatic memory management.
Programming Language | Memory Management Approach | Key Considerations |
---|---|---|
C | Manual | Requires careful handling of memory allocation and deallocation to avoid memory leaks and other issues. |
Java | Automatic (Garbage Collection) | Simplifies development but can introduce unpredictable behavior and performance challenges. |
Python | Hybrid (Automatic and Manual) | Offers a balance of convenience and control, with the ability to leverage both automatic and manual memory management techniques. |
Understanding how each language manages memory helps programmers make better choices. This leads to more efficient and reliable software development.
Emerging Trends in Memory Technologies
The world of computer memory is always changing. New and innovative technologies are coming up, changing how programmers make and improve their apps. These new memory types and technologies are amazing.
New Memory Types and Their Advantages
New memory types are exciting in the memory tech world. They aim to fix the problems of old memory types. These new solutions offer big benefits for programmers and their apps.
Some of the new memory types include:
- Persistent Memory – It’s fast like RAM but keeps data even when turned off, making it a bridge between RAM and storage.
- Magnetic RAM (MRAM) – This memory doesn’t lose data and is as fast as RAM, making it a good choice.
- Resistive RAM (ReRAM) – It stores data by changing resistance, which could mean faster speed, less power use, and more room to grow.
- Phase Change Memory (PCM) – Using special materials, PCM offers better performance and lasts longer than old memory types.
These new memory technologies aim to meet the need for more storage, faster data access, and less power use in computers. Programmers using these new types can make their apps run better, grow bigger, and be more reliable.
Memory Type | Key Advantages |
---|---|
Persistent Memory | Fast data access, non-volatile data retention |
Magnetic RAM (MRAM) | Speed, endurance, non-volatile storage |
Resistive RAM (ReRAM) | Speed, power efficiency, scalability |
Phase Change Memory (PCM) | Performance, endurance, scalability |
As memory technology keeps changing, programmers who keep up and use these new trends will be ahead. They can make apps that use the best of these new memory types.
“The evolution of memory technology is a critical driver of progress in the computing industry. As new memory types emerge, programmers have the opportunity to unlock unprecedented levels of performance, efficiency, and versatility in their applications.”
Memory Security and Vulnerabilities
In software development, memory security is key. Programmers must know about common security issues from bad memory management. These issues can let hackers break into software systems.
A big worry is the buffer overflow vulnerability. This happens when a program writes too much data into a buffer. This can let hackers run their own code or see private data.
Another issue is the use-after-free vulnerability. This is when a program uses memory that’s already been given back. Hackers can use this to control the program or the whole system.
- Buffer overflows can let hackers run their own code or see private data.
- Use-after-free vulnerabilities can be used to control a program or the system.
- Memory leaks can cause system crashes and denial-of-service attacks.
To fix these issues, developers need to use many strategies. They should check inputs, use dynamic memory, and find memory leaks. Also, choosing memory-safe languages and compiler security features helps a lot.
“By understanding the common memory-related security vulnerabilities and employing best practices, developers can create more secure software that is less susceptible to malicious attacks.”
Memory security is very important in making software. By fixing these problems, developers can make apps that protect users’ data and keep systems safe.
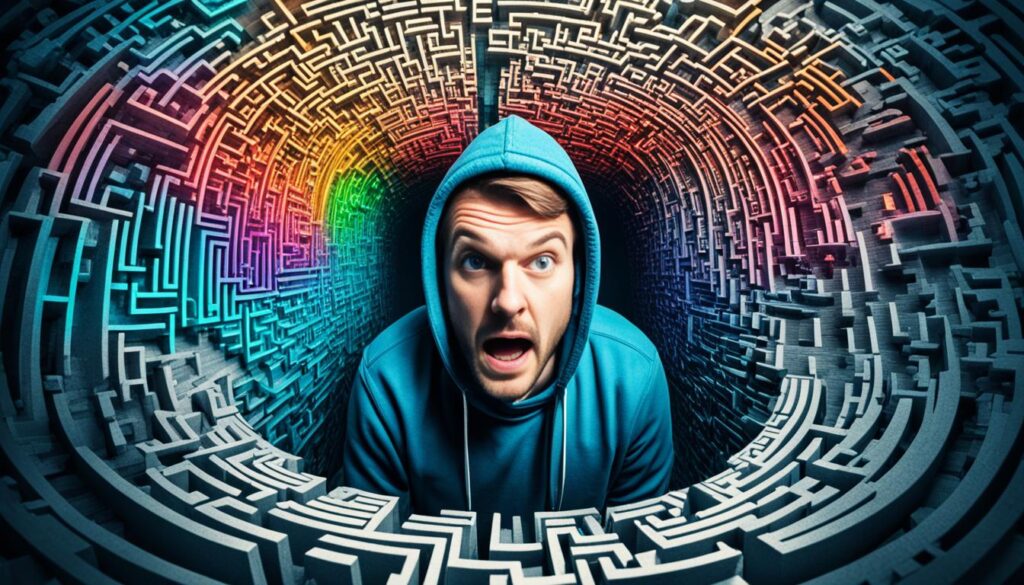
Conclusion
We’ve explored memory concepts for programmers in depth. We now understand what every software developer needs to know. Topics like RAM, ROM, memory management, optimization, and security are key for making strong apps.
We learned about static and dynamic memory allocation. We also looked at the heap and stack’s roles. Memory leaks and their effects were discussed, along with the importance of cache memory and virtual memory. We touched on new trends in memory technologies too.
Knowing about memory helps programmers make their code better and faster. It also helps protect against security risks. This knowledge lets developers create apps that are efficient with memory and can handle new challenges. As memory technology changes, staying updated is crucial. But the basics we covered will help programmers get started on the right path.
FAQ
What are the different types of computer memory?
Computer memory includes RAM and ROM. RAM loses data when turned off, while ROM keeps data even without power.
What is the difference between static and dynamic memory allocation?
Static memory allocation sets memory size at compile-time. Dynamic allocation lets programs allocate and free memory at runtime, making memory use more flexible.
How does the heap and stack memory work?
The heap and stack are memory regions with different roles. The stack stores local variables and function info, while the heap is for dynamic memory. Knowing their roles helps manage memory well.
What is virtual memory, and how does it work?
Virtual memory acts as a bridge between programs and physical memory. It uses paging and segmentation to let programs use more memory than available, storing data on disk when not in use.
How can programmers identify and prevent memory leaks?
Memory leaks happen when memory isn’t released, causing a program to use more memory over time. Tools for memory profiling and debugging help find and fix these leaks.
What is the role of cache memory, and how can it be leveraged for performance optimization?
Cache memory is fast memory that helps access data quickly. By understanding how data is stored in cache, programmers can write code that uses cache efficiently, improving performance.
What are some common memory optimization techniques?
To optimize memory, use the right data structures, design efficient algorithms, and minimize memory use. Using language features for memory management also helps improve performance.
How can programmers use memory profiling and debugging tools?
Tools like memory analyzers and leak detectors help find and fix memory issues. They show how memory is used, helping programmers write more efficient code.
What are some common memory-related security vulnerabilities?
Issues like buffer overflows and dangling pointers can cause serious security problems. Programmers should know these risks and follow secure coding practices to protect against them.