Functions and procedures are key parts of programming. They are small, reusable pieces of code that do one specific job. Programmers use them to avoid repeating commands and to make programs easier to understand.
They break down big programs into smaller, easier parts. This makes it simpler to manage and improve the code.
Functions and procedures make it easier to work with data and organize code. They make the code clear and easy to read. This helps with debugging and testing, making the software better and more reliable.
By using functions and procedures, programmers can make their work more efficient. They can write code that is easy to understand and use. This leads to programs that are easier to maintain and can grow with new needs.
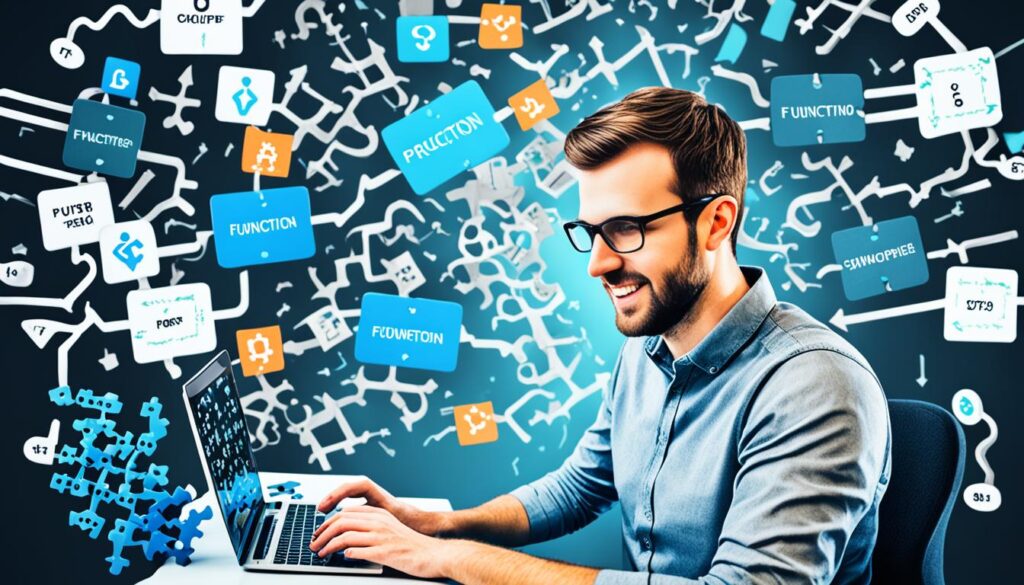
Key Takeaways
- Functions and procedures help avoid repeating commands, reducing program size.
- Implementing functions and procedures makes the code easier to maintain.
- Use of functions and procedures creates a logical structure by breaking down programs into smaller modules.
- Functions return a value, while procedures do not (a function is a procedure that returns a value).
- Variables declared within functions or procedures are local and can only be used within that function.
Introduction to Functions and Procedures
In computer programming, functions and procedures are key tools. Programmers use them to make their code better and easier to understand. These tools help build software that is organized, easy to keep up with, and can grow.
Understanding the Purpose of Functions and Procedures
Functions and procedures wrap up a certain task in a program. They let programmers turn big programs into smaller, easier parts. This way, complex programs become simpler to grasp, keep up with, and grow.
The Importance of Modular Programming
Modular programming is all about using functions and procedures. It’s a key idea in making software. By breaking a program into smaller, independent parts, programmers make the code better, more reusable, and easier to test and fix.
This way, the code is clearer and easier to keep up with. Each part of the code has its own job, making everything more organized.
“Modular programming is the process of breaking a program into distinct features and functions, which can then be independently created, tested, and integrated.”
Code Reusability
Avoiding Repetition in Code
Using functions and procedures in programming helps avoid repeating code. If some operations are needed in different parts of an app, it’s smart to make a function or procedure. This way, you don’t copy the same code over and over. It makes the program smaller and easier to keep up with, since changes are made in one spot.
Internal reuse means using code made by a team or business in other projects. External reuse is using code from others that you’ve licensed. Both types save time by making it so you don’t have to write the same code again. Code reuse makes developers more productive and lets them work on new stuff.
Using code that’s already made cuts down on costs and risks in app development. It uses code that’s already proven to work well. Code reuse also keeps code simple and efficient, avoiding too much complexity.
In a microservices setup, code reuse lets different teams use the same code in their services. This boosts productivity in making software. But, it can be hard to communicate and manage as projects get bigger, and there might be office politics and the work to keep code libraries up to date.
“Before reusing code, quality must be assessed by evaluating security, reliability, performance efficiency, and maintainability. Testing code prior to reuse is crucial to ensure functionality; tools like Browserstack offer testing on various device and browser combinations for seamless manual or automated tests.”
Code reusability lets developers save time on repetitive tasks and make code maintainability better. This makes software development more efficient overall.
Modular Programming and Abstraction
Modular programming has been key in software development since the 1960s. It breaks down big programs into smaller, easier-to-handle modules. Each module has its own job and set of tasks.
At the core of modular programming is data abstraction. This means using functions and procedures to keep data safe and hide how it’s stored. It makes programming easier to understand and maintain by organizing it into smaller, clear modules.
This way of organizing code has many benefits. It makes the program easier to get, fix, and keep up with over time. TinyMCE at Tiny shows how their modular setup keeps files short, unlike big apps with thousands of lines. This makes the code easier to read.
Modular programming also makes code reusable. Functions and procedures can be used in different parts of the program. This cuts down on code duplication and the chance of mistakes. It also makes testing easier, as modules can be checked one by one, and changes are safe under the API layer.
“Modular programming divides a computer program into separate sub-programs or modules, each of which addresses a specific task or responsibility.”
In short, modular programming, with its use of functions and procedures, is a key practice. It improves organization, readability, reusability, and maintainability of code. By abstracting data and breaking tasks into smaller modules, programmers can make software better and more efficient.
Code Organization and Readability
For programmers, making code easy to read and maintain is key. Breaking a program into smaller parts helps a lot. Using functions and procedures makes the code clear and easy to work with. This way, it’s simpler for others to join in and understand the code.
Functions and procedures help organize code into easy-to-manage pieces. They make it easier to focus on one part at a time. This approach also makes the code simpler to read and change later.
Functions have a clear name that tells what they do, like “makeFries”. They take in information through parameters and give back a result with a return statement. Calling a function means using its name and giving it the needed values.
It’s important to keep track of what a function needs and what it does. Following rules like keeping lines short makes the code easier to read. This helps with code organization and code readability.
Breaking code into modules and functions makes the program’s structure better. Putting math-related functions in a single module makes things clearer. Turning repeated tasks into reusable functions cuts down on repetition and boosts code reusability.
Benefit | Description |
---|---|
Improved Structure | By splitting code into modules and functions, the overall structure of the program improves. |
Logical Organization | Grouping functions related to mathematical calculations in the utils.py module enhances code organization. |
Redundancy Reduction | Refactoring repetitive calculations into reusable functions eliminates the need to repeat calculations, reducing redundancy. |
Code Reusability | Encapsulating calculations within functions promotes code reusability and saves development time. |
Accuracy | Descriptive function names accurately represent the purpose of each function, enhancing code comprehension. |
Single Source of Truth | Refactoring repetitive tasks into reusable functions ensures a single source for calculations, improving maintainability. |
In conclusion, using functions and procedures is key to better code organization and code readability. This supports the modular programming approach and makes software projects easier to maintain.
Debugging and Code Testing
Debugging and code testing are key parts of making software. Programmers use functions and procedures to make these steps better and more effective. By testing each part of the code, developers can fix problems faster and make sure everything works right.
Isolating and Testing Code Modules
Functions and procedures let programmers isolate and test code modules on their own. They don’t have to test the whole program at once. This way, they can find and fix issues early and understand where problems come from.
Good debugging and code testing use many techniques, like logging and tracing. By watching how each module works, programmers can spot and fix errors quickly. This makes the software better quality.
“Debugging is a cyclic activity involving execution testing and code correction. Implementation testing involves not only execution testing but also correctness proofs, code tracing, and peer reviews.”
Peer reviews are important too, even though they take time. When different developers check the code, they can find mistakes that one person might have missed.
The ability to isolate and test code modules is a big plus of using functions and procedures. This method makes debugging and testing easier, leading to better software that’s easier to keep up with.
Maintainability and Scalability
The use of functions and procedures is key in programming. It makes software easier to maintain and scale over time. By breaking the code into smaller parts, programmers can fix problems and add new features easily. This modular programming way helps the software grow without breaking.
Good algorithms are crucial for code scalability. They make data processing faster and smoother. This is vital as software often needs to handle more users and data.
Modular programming also makes code reusability and maintainability better. It puts tasks and functions into separate parts. This makes it simpler to update or fix parts without affecting the whole code.
“Scalability is the capacity of a system to adjust performance and costs in response to changes in processing needs, ensuring that applications can handle increased workload with low development expenses.” – Gartner
To make software scalable, developers use cloud hosting for flexibility. They also use load balancing, caching, APIs, and asynchronous processing. These methods help handle more users and tasks efficiently.
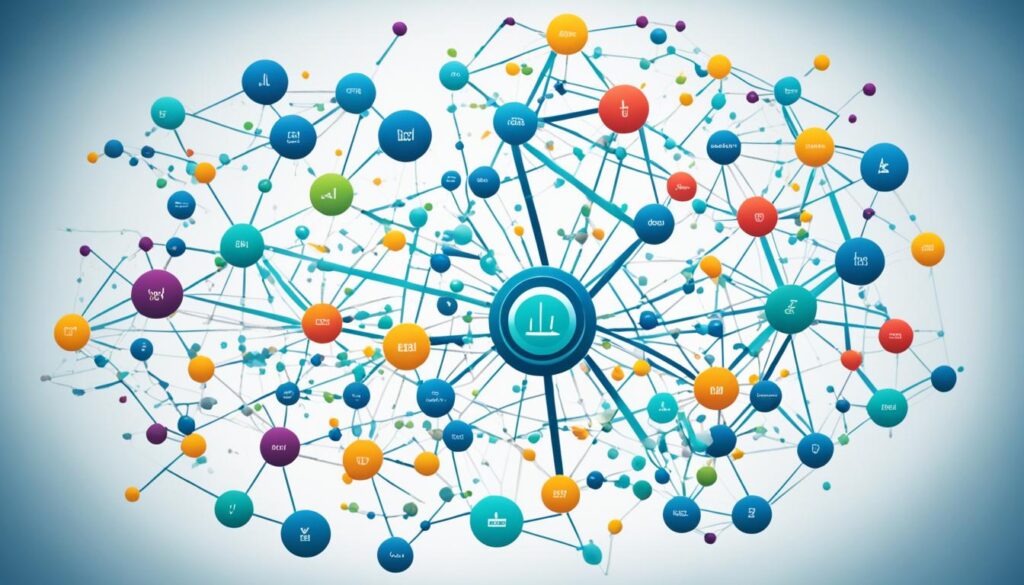
Using functions and procedures helps make software strong and flexible. It’s not just good for now but also for the future. This approach makes software that can grow and change as needs do.
Functions as Building Blocks
Programmers use functions and procedures as the basic parts to make complex software. These parts do specific tasks and make big tasks smaller. This way, it’s easier to understand and improve the code over time.
Breaking Down Complex Tasks
Functions help hide the complex parts of a problem, letting programmers focus on each part. This makes the code easier to work with, test, and grow as needs change.
In F#, developers use the “>>” operator to link simple functions into complex ones. This makes the code easier to read and shorter. In JavaScript, functions help organize tasks and make clear how inputs turn into outputs.
Using functions has more than just organizational benefits. In F#, combining functions easily creates new powerful actions. Using DSLs in F# makes code clearer for certain tasks.
Functions in F# are very flexible and can be easily changed to add new features. This is different from OOP, which focuses on making interfaces through functions and methods.
Functions are key to modular programming. They help break down big tasks, make code easier to read, and make software better over time.
why programmers use functions and procedures ?
Encapsulating Tasks and Improving Code Clarity
Programmers use functions and procedures to make their code clear and organized. They break down big tasks into smaller, easy-to-understand parts. This makes the code easier to read and maintain.
It also makes it easier to use the same code in different parts of the program. This cuts down on code duplication and makes the program run better.
Functions let programmers return values, making the code more efficient and reusable. Procedures are like reusable blocks of code that can be used over and over. Breaking down big tasks into these smaller parts helps improve programming skills.
Using local variables in functions helps avoid mistakes and makes the code easier to read. Python functions can return different types of data and take in user input. This makes the code more flexible and customizable.
Benefit | Explanation |
---|---|
Encapsulating Tasks | Functions and procedures help programmers focus on specific tasks. This makes the code clearer and easier to understand. |
Code Reuse | Using functions and procedures lets the same code be used in different parts of the program. This reduces code duplication and makes the program run smoother. |
Improved Readability | Breaking code into smaller units makes it easier to read and maintain. Functions and procedures help with this. |
“Functions in programming are likened to functions in mathematics with an input and output requirement, whereas procedures can run code without the need for inputs or outputs.”
In summary, programmers use functions and procedures to make their code clear and organized. This approach makes the code reusable, readable, and easier to maintain. It leads to more efficient and scalable software development.
Function Workspace and Variable Scope
In programming, each function has its own workspace. This means variables used in a function can only be accessed within that function. This rule helps avoid mistakes that could change variables in other parts of the program.
This way, you can use the same variable name in different modules without problems. It makes the code easier to work with and maintain. Developers can focus on one part of the software without worrying about the rest.
In MATLAB, variables are kept in separate areas of memory called workspaces. The Base Workspace is for the command-line, and the Global Workspace is for global labels. Use these labels carefully to avoid name clashes. MATLAB functions also have their own workspace, called the function scope.
Also, MATLAB lets you use recursive functions. These are functions that call themselves to solve a problem. Each recursive call has its own set of variables, keeping the code clear and organized.
Knowing about function workspaces and variable scope helps developers write better code. It makes the code easier to understand, maintain, and expand. This leads to better quality and success in programming projects.
Workspace Type | Description |
---|---|
Base Workspace | Created for the command-line environment in MATLAB |
Global Workspace | Reserved for global labels, which should be used sparingly to avoid name collisions |
Function Scope | The workspace specific to a MATLAB function, where variables are only accessible within that function |
“Functions in MATLAB contain one or more statements that implement an algorithm. Variables in MATLAB are organized into separate segments of memory called a workspace.”
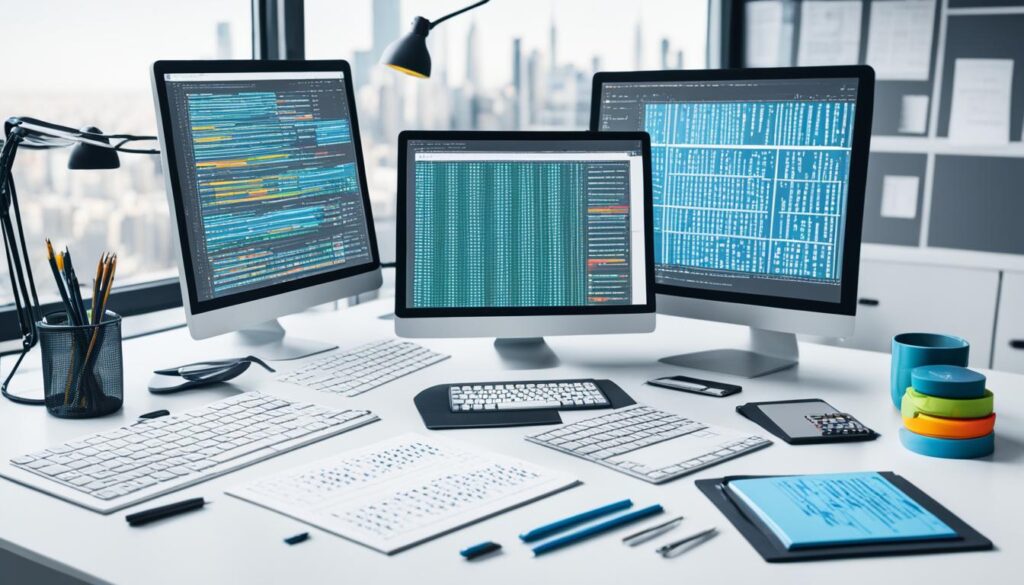
Formal and Actual Parameters
Programmers use formal parameters as placeholders for data in functions or procedures. These are swapped with actual parameters, which are the specific values used when the function is called. This setup makes the code more flexible and reusable, allowing it to work with different data without changes.
Formal parameters are the variables listed in a function’s parameter list. They must have a data type. Actual parameters are the values given when a function is called. They don’t need a data type to be defined.
The main differences between formal and actual parameters are:
- Formal parameters are in the function being called, while actual parameters are in the calling function.
- Formal parameters need a data type, but actual parameters don’t.
- Formal parameters act like local variables in the function. Actual parameters can be constants or variables.
- Actual parameters are used in function calls and are called arguments. Formal parameters are defined in the function.
Knowing about formal and actual parameters is key in programming languages like C. It helps write efficient, reusable code. By using these parameters, programmers can make their code modular, easy to maintain, and adaptable to various situations.
“Passing data to functions is a fundamental concept in programming that allows for code reuse and abstraction. Mastering formal and actual parameters is key to writing flexible, maintainable, and scalable code.”
Function Nesting and Utilization
In programming, functions and procedures are key to making software better. They can’t be nested, but you can call other functions inside them. This lets you build complex programs by using the strengths of different functions and procedures.
Using functions this way makes software more modular and scalable. Functions group many instructions into one line, making code simpler. Most languages have built-in functions to make tasks easier. They also let you reuse code, so you don’t have to write the same thing over and over.
Calling other functions within a function is a strong technique. It turns complex programs into a series of simpler steps. Functions keep variables clear by limiting their scope to the function they’re in. This keeps the code organized and prevents mistakes.
Even though you can’t nest functions, you can call them inside another function. Many languages like ALGOL, Simula 67, and Pascal support this. It can lead to closures, which affect how the code works and performs.
Programming Language | Nested Function Support |
---|---|
ALGOL, Simula 67, Pascal | Supported nested functions |
Scheme, JavaScript, Dart, Kotlin, Rust, Scala | Commonly support nested functions |
Ruby, Python, Lua, PHP, Perl | Varying degrees of nested function support |
C# | Adopted nested functions starting with C# 7.0 |
D, Rust, MATLAB | Fully support nested functions |
Function nesting and function utilization in modular programming make code better. They help programmers handle complex tasks by breaking them into smaller parts.
Real-Life Analogies and Examples
Brushing Teeth as a Procedure
Real-life analogies help us understand programming better. A good example is brushing your teeth. This simple task has several steps that together make a complete process.
To brush your teeth, you follow a specific order:
- Grab your toothbrush
- Squeeze toothpaste onto the bristles
- Brush all your teeth, front and back
- Rinse your mouth with water
- Put away your toothbrush and toothpaste
These steps, though simple, form a procedure to clean your teeth. This is similar to how programming uses functions and procedures for specific tasks. The “brushing teeth” process shows how modular programming works in real life.
When you brush your teeth, you don’t start from scratch. You recall the “brushing teeth” procedure and follow it. This is the same idea that programmers use in their code. They design functions and procedures to be modular and reusable.
“The next time you need to brush your teeth, you remember the ‘brushing teeth’ procedure and know exactly what to do, much like how programmers use functions and procedures to encapsulate and reuse specific tasks within a program.”
Comparing everyday tasks with programming helps us see the value of real-life analogies, brushing teeth as a procedure, and modular programming. This makes software development clearer.
Functions in Different Programming Languages
Functions and procedures are key in most programming languages, like JavaScript, Visual Basic, and Python. They help with modular programming, code reuse, and abstraction. Even though names and some details change, the core ideas stay the same across languages.
In JavaScript, you make a function with the function
keyword. You add the function name, parameters, and the code in curly braces. These functions can give back values or just do tasks.
Visual Basic has functions and subroutines. Functions give back values, and subroutines don’t. You declare them differently, using Function
for functions and Sub
for subroutines.
Python uses functions too, which are made with the def
keyword. They can return values or do actions. Python functions can also have default values for optional parameters.
Programming Language | Functions | Procedures/Subroutines |
---|---|---|
JavaScript | Defined using the function keyword, can return values | N/A (everything is a function in JavaScript) |
Visual Basic | Defined using the Function keyword, return values | Defined using the Sub keyword, no return value |
Python | Defined using the def keyword, can return values | N/A (everything is a function in Python) |
Functions and procedures are key in programming language features. They help make software design better and more modular.
“Functions and procedures are the building blocks of modular programming, allowing developers to break down complex tasks into manageable, reusable components.”
Conclusion
Programmers use functions and procedures to make code easier to use again, more organized, and easier to read. They also make debugging and testing simpler. This helps create software that is easy to keep up with and can grow.
By breaking down big programs into smaller parts, programmers can handle the work better. This makes working together, finding problems, and keeping the software up to date easier. Functions and procedures are key to modern programming. They help developers make strong and flexible software solutions.
Functions and procedures have many benefits. They make the code organized, reusable, easier to keep up with, and simpler to test and debug. Functions handle specific tasks, and procedures do several tasks in one block of code. This helps developers write applications that are clean, modular, and can change easily.
Using functions and procedures well is a sign of good software development. It leads to a more organized, efficient, and lasting way of making complex systems. By using these techniques, developers can make software that is reliable, easy to maintain, and meets the changing needs of users and the business.
FAQ
Why do programmers use functions and procedures?
Programmers use functions and procedures to avoid repeating commands in their code. They break down the program into smaller parts. This makes the code easier to understand and maintain.
What is the purpose of functions and procedures?
Functions and procedures help programmers tackle complex tasks by breaking them into smaller steps. They make the code easier to read and maintain.
How do functions and procedures contribute to code reusability?
Functions and procedures help avoid code repetition. By using a single function or procedure in different parts of the program, the code becomes shorter and easier to manage.
How do functions and procedures facilitate modular programming and abstraction?
Functions and procedures make programming modular. This approach helps in abstracting data-storage functions. It makes the program easier to understand and maintain over time.
How do functions and procedures improve code organization and readability?
By dividing the program into smaller, focused tasks, programmers can make their code easier to read. This makes it simpler for others to understand and work with the codebase.
How do functions and procedures contribute to debugging and testing?
Functions and procedures help in debugging and testing by allowing programmers to test individual modules. This approach helps catch issues early and ensures each part of the program works correctly.
How do functions and procedures enhance the maintainability and scalability of a program?
Using functions and procedures makes a program easier to maintain and scale over time. It breaks the code into smaller modules. This makes it simpler to fix issues and add new features.
How do functions and procedures serve as the building blocks of programs?
Functions and procedures are the building blocks of programs. They allow programmers to break complex tasks into smaller steps. This makes it easier to focus on individual parts of a problem and put them together into a complete program.
How do functions and procedures improve code clarity and program structure?
Functions and procedures improve code clarity by encapsulating specific tasks. This makes the code easier to understand and maintain. It helps in organizing the program structure better.
How do function workspaces and variable scope contribute to software engineering?
Function workspaces and variable scope are key in software engineering. They prevent changes to variables that could affect other parts of the program. They also allow for the reuse of variable names across different modules.
How do formal and actual parameters work in functions and procedures?
Formal parameters are placeholders in function definitions. Actual parameters are the values or variables used when calling the function. This separation allows for flexibility and reusability, making the same function work with different data without changing the code.
Can functions and procedures be nested, and how do programmers utilize them?
Functions and procedures can’t be nested, but they can be called within each other. This allows for creating complex and robust programs. It lets the functionality of a module build on the capabilities of other specialized functions and procedures.
Can you provide a real-life analogy for the use of functions and procedures?
Brushing your teeth is like a procedure. It involves steps like getting the toothbrush, applying toothpaste, brushing, rinsing, and putting things back. These steps are part of the ‘brushing teeth’ procedure. Programmers use functions and procedures similarly, encapsulating specific tasks to make programming easier and more efficient.
How are functions and procedures implemented in different programming languages?
Functions and procedures are key in many programming languages, like JavaScript, Visual Basic, and Python. They help with modular programming, code reuse, and abstraction. The syntax may vary, but the core principles stay the same across languages.