Programmers solve problems by turning information into steps. Computers work with only a few types of data. Programmers use variables and data structures to “encode” the problem’s facts. Algorithms are key in solving problems, but computers can only do a few basic things. So, programmers break big problems into smaller ones.
Programmers use many methods to make problems easy for computers. They use formal languages and knowledge methods to make models and structures. These help capture the problem’s key parts.
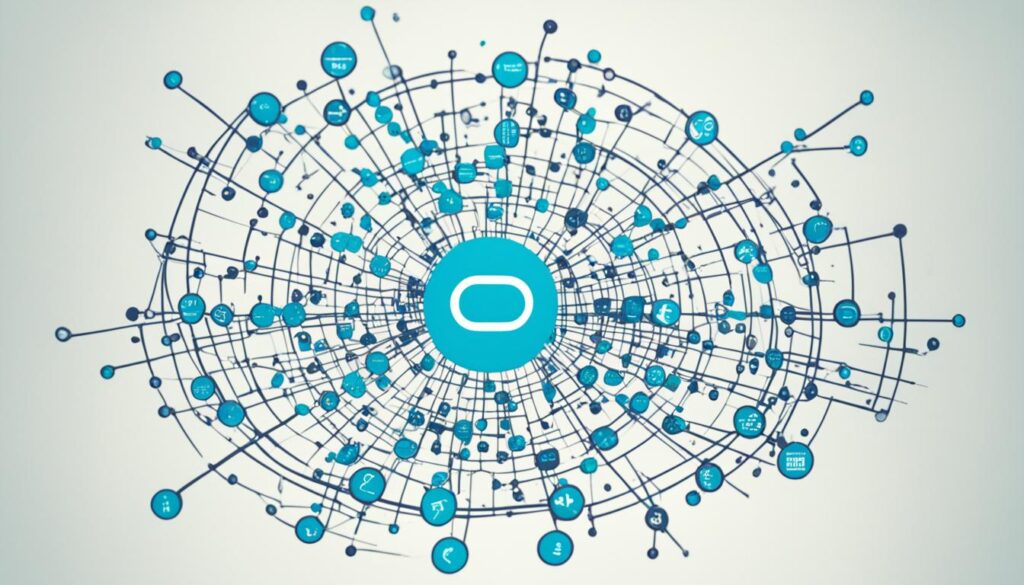
Key Takeaways
- Programmers must represent problems and systems in a way that computers can understand, using data types, algorithms, and computational models.
- Formal languages, knowledge representation techniques, and symbolic reasoning are key tools for encoding information and creating effective computational representations.
- Breaking down complex problems into smaller, more manageable sub-problems is a crucial skill for programmers to develop.
- Algorithms, which are step-by-step procedures for solving a problem, are at the heart of programming and problem-solving.
- Mastering abstraction and complexity management is essential for programmers to tackle increasingly sophisticated problems and systems.
Understanding the Problem
Mastering problem-solving is key for programmers. It’s vital to understand the problem before solving it. This means explaining it in simple language, without using hard technical terms.
Explain the Problem in Plain English
Breaking the problem down into simple terms helps programmers identify the key elements to fix. They look at inputs, outputs, and what the solution needs to do. This clear definition is the first step to finding a solution.
Identify the Key Elements
Good programmers can break down complex problems and look at them from different sides. This helps them find the problem’s core and what it needs. Learning to analyze problems well is key for programmers.
“Demonstrating computational thinking or the ability to break down large, complex problems is highly valued by employers.”
By focusing on problem understanding, explanation, and identification, programmers set up for success. This method improves their problem-solving skills and overall programming abilities.
Planning the Solution
Effective solution planning is key for programmers. It means outlining the steps to turn inputs into the desired outputs. This creates a roadmap that helps implement the solution and makes sure it meets the needs.
Outline the Steps
First, break the problem into easy steps. This includes:
- Identifying the key inputs and outputs
- Determining the necessary data transformations or calculations
- Organizing the steps in a logical sequence
- Considering potential edge cases or special circumstances
- Estimating the time and resources required for each step
Use Comments for Clarity
Adding clear and concise code comments helps with the problem-solving process. These comments explain the purpose and functionality of each step. This way, programmers can:
- Enhance the readability and maintainability of the code
- Facilitate collaboration and code sharing with other developers
- Simplify the debugging and troubleshooting process
- Ensure the solution is easily understood and updated in the future
By planning the solution well and using comments for clarity, programmers can make solving complex problems more efficient. This approach improves the problem-solving process overall.
“Proper planning and preparation prevent poor performance.” – Keller Williams
Divide and Conquer
Programmers often use a “divide and conquer” approach to tackle complex problems. Instead of solving the whole problem at once, they break it down into smaller parts. This makes the problem easier to solve, debug, and test.
Break Down into Sub-Problems
The first step is to break the problem into smaller, easier parts. This process is called problem decomposition. It means dividing the problem into parts that can be solved on their own.
- Analyze the problem and identify the distinct components or sub-tasks that need to be addressed.
- Determine the relationships and dependencies between the sub-problems.
- Assign priorities and decide the order in which the sub-problems should be solved.
Solve Sub-Problems Incrementally
After breaking down the problem, programmers focus on solving each part incrementally. This means they work on smaller tasks and build a solution step by step.
- Solve each sub-problem independently, using appropriate algorithms and techniques.
- Test and verify the correctness of the solutions for the sub-problems.
- Integrate the solutions to the sub-problems into a complete solution for the original problem.
By dividing and conquering, programmers can turn complex problems into smaller, easier parts. They solve these parts step by step and then combine the results to find a solution.
Algorithm | Time Complexity | Description |
---|---|---|
Merge Sort | O(n log n) | A divide-and-conquer sorting algorithm that works by recursively dividing the input array into smaller subarrays until they are small enough to sort. |
Strassen’s Matrix Multiplication | O(n^2.8074) | A divide-and-conquer algorithm for multiplying two matrices, which is faster than the standard matrix multiplication algorithm. |
Cooley-Tukey Fast Fourier Transform (FFT) | O(n log n) | A divide-and-conquer algorithm used to compute the discrete Fourier transform, which is an important tool in signal processing and many other fields. |
Debugging and Troubleshooting
Even the best programmers run into problems and bugs in their code. When you hit a roadblock, it’s important to be curious and open to trying new things. Programmers use step-by-step debugging to find where the code is failing. They’re also not afraid to reassess their approach if needed. This way of solving problems is key to getting past hurdles.
Step-by-Step Debugging
Debugging means going through the code step by step to find and fix issues. Programmers begin by reproducing the problem to get to the bottom of it. They use tools like breakpoints and logging to pinpoint the exact location of the bug. This careful method helps them isolate and address the issue well.
Reassessing the Approach
If the first tries at debugging don’t work, successful programmers are ready to reevaluate their strategy. They might need to redefine the problem, look at new solutions, or even try a completely different approach. Being flexible and open to change is key in solving tough debugging and troubleshooting problems.
“The best programmers are not the ones who never encounter bugs, but the ones who are relentless in their pursuit of solutions.”
By using a step-by-step debugging process and being open to problem reassessment, programmers can tackle even the toughest debugging and troubleshooting challenges. This approach, along with the right tools and methods, is what helps find solutions and create reliable software.
Research and Learning
Successful programmers always keep learning new things. They dive into new techniques, algorithms, and technologies to get better at solving problems. They don’t just copy solutions; they try to understand the reasons behind them. This constant drive for continuous improvement and problem-solving learning sets them apart.
J.M. Wing introduced the idea of computational thinking in 2006 through an essay in the Communications of the ACM. But, the idea of procedural thinking was talked about by S. Papert ten years before that. Now, computational thinking is seen as a key skill in many fields, from science to art.
Computational thinking includes skills like decomposition, pattern recognition, abstraction, and algorithmic thinking. Since 1999, Learning.com has been a leader in teaching digital skills, including coding and computational thinking, to students in grades K-12.
Computational Thinking Components | Description |
---|---|
Decomposition | Breaking down a complex problem into smaller, more manageable sub-problems. |
Pattern Recognition | Identifying similarities and differences in data to uncover underlying structures. |
Abstraction | Focusing on the essential features of a problem and ignoring irrelevant details. |
Algorithmic Thinking | Developing a step-by-step procedure to solve a problem. |
Many people have wrong ideas about memory and learning, showing how crucial continuous learning and using evidence is for problem-solving. By always doing programming research and problem-solving learning, programmers can lead in their field and help advance technology.
Data Representation
Programming is all about how we represent and work with data. Programmers use variables, data types, and structures to encode the problem’s information. The way we represent data greatly affects how well and efficiently we solve problems.
Encoding Information
Information encoding is key in data representation. Computers use only two digits: 0 and 1, in binary data. To work with text, like letters and symbols, we use character encoding systems like ASCII and Unicode.
These systems give each character a unique number. This lets computers understand and work with text.
Data Types and Structures
Choosing the right data types and data structures is important. Data types tell us what kind of values a variable can hold, like numbers or letters. Data structures help organize data in ways that make it easier to work with, like lists or trees.
Picking the right data types and structures is key. It affects how well a program works, how much memory it uses, and its overall design. Knowing how to use these basics is a key skill for programmers.
“The way data is represented can have a significant impact on the efficiency and effectiveness of the solution. Understanding how to choose the appropriate data types and structures is a crucial skill for programmers.”
Algorithms and Logic
Programming is all about the complex world of algorithms and logic. Algorithms are like step-by-step guides for solving problems. They are key in computer science. Programmers need to know how to use these algorithms and think like computers to solve complex problems.
Step-by-Step Procedures
Algorithms are vital in many fields, like manufacturing, healthcare, finance, and transportation. In manufacturing, they help make production better and cut down on waste. In finance, they help with analyzing data to make smart investment choices.
Healthcare uses algorithms for things like processing medical images, diagnosing diseases, and improving treatment plans.
Computational Thinking
Computational thinking is what makes algorithms powerful. It’s about breaking down big problems into smaller ones, finding patterns, and solving them step by step. This way, programmers can make algorithms that work well and can grow with technology.
Learning about algorithms and computational thinking is a must for programmers. These skills help solve a wide range of problems, from simple to complex. As technology gets more advanced, the need for skilled programmers will keep growing.
“Algorithms are the lifeblood of the digital age, powering everything from search engines to recommendation systems. Mastering their inner workings is crucial for any programmer who wants to create truly innovative solutions.”
Encapsulation and Abstraction
Programmers often struggle with the complexity of software systems. Encapsulation and abstraction are key to tackling this issue.
Complexity Hiding through Encapsulation
Encapsulation wraps up data and functions into single “objects.” This way, programmers can keep the inner workings hidden. It makes the system simpler and keeps data safe by hiding its inner details.
In a C++ program, making a variable private and only accessible through certain class methods is an example of encapsulation. This keeps the inner workings safe, protecting the data and the system’s integrity.
Abstraction: Focusing on the Essentials
Abstraction picks out the main features and actions of a system or object, leaving out the less important parts. This makes the code simpler and easier to read. It often uses abstract classes and interfaces to focus on what’s really needed without getting into the details.
Take a look at a C++ code snippet that shows how abstraction simplifies things. It demonstrates how to make the code simpler and hide the complex parts.
Abstraction and encapsulation are different. Abstraction looks at the design or interface level, while encapsulation looks at the inner workings. Abstraction hides information, and encapsulation keeps data in one place.
Using encapsulation and abstraction helps programmers deal with complexity. It makes code more reusable and modular. This leads to better software that’s easier to maintain and grow.
Pattern Recognition
As programmers, recognizing patterns is key. It helps us find common elements and themes in problems. This lets us create solutions that work across different situations. Pattern recognition helps us see trends and make complex things simpler.
Identifying Common Elements
First, we look closely at the problem to find common elements. This might mean spotting similar data structures or algorithms. By finding these common elements, we can understand the problem better and solve it more efficiently.
Describing Patterns
After identifying elements, we describe the pattern clearly. This could mean making a mental model or writing down best practices. By sharing our patterns, we help others solve problems too.
Pattern Recognition in Action | Key Statistics |
---|---|
80% of dataset used for training, 20% for testing in pattern recognition systems Feature vectors represent key attributes in pattern recognition models Accuracy of 70% in flower recognition example k-NN algorithm finds similar instances in pattern recognition applications Pattern recognition used in fingerprint identification, image processing, and more | Computational thinking involves decomposition, pattern recognition, abstraction, and algorithmic thinking Pattern recognition simplifies problems and enhances comprehension of complexities Pattern recognition aids in predicting outcomes, diagnosing diseases, and creating common solutions |
Mastering pattern recognition boosts efficiency and creativity in solving problems. It helps us spot and describe patterns, whether in design, data, or complex systems. This skill is a key asset for programmers.
“Patterns simplify our understanding of the world and enable us to focus on the essential aspects of a problem, rather than getting bogged down in the details.”
Pattern Generalization and Abstraction
Programmers often do more than just spot patterns. They learn to generalize and abstract these patterns. This lets them use solutions on a wider range of problems. They find the core principles of a solution and express them in simpler terms.
Generalization means finding common elements in related problems or solutions. Skilled programmers can spot recurring themes and adapt them for new challenges. This skill helps them solve new problems more efficiently.
Abstraction simplifies complex problems by focusing on the key features and relationships. Programmers use this skill to create general solutions that work in many situations. They remove details that aren’t crucial.
These skills, pattern generalization and pattern abstraction, are key for solving problems effectively. They help programmers use a set of solutions for many problems, instead of starting over each time.
- Identify the core principles and structures of a solution
- Generalize the solution to make it broader in application
- Abstract the solution by removing what’s not essential
- Use the generalized and abstracted solution on new problems
Skill | Description | Example |
---|---|---|
Pattern Generalization | Identifying common elements and structures within a set of problems or solutions | Recognizing that different sorting algorithms, such as bubble sort, merge sort, and quicksort, share the underlying principle of comparing and rearranging elements to put them in order |
Pattern Abstraction | Simplifying complex problems by focusing on the essential features and relationships | Representing a complex data structure, such as a binary tree, using a high-level diagram that captures the key nodes and their relationships, without getting bogged down in implementation details |
“Abstraction is one of the key principles of computational thinking. It involves removing unnecessary details from a problem, focusing on the core information needed to solve it.”
General Representation
Developers need to think about the main parts of a problem or system. This includes variables, which change, and constants, which stay the same. It’s also key to know the inputs and outputs of a problem or system.
Variables and Constants
Variables change during a program’s run, storing and changing values. Constants don’t change, staying the same from start to end. Knowing and managing these parts is vital for making strong, dependable software.
Inputs and Outputs
The inputs are what you put into a program, and the outputs are what you get out. Knowing these helps programmers understand the problem, design better algorithms, and make sure the program works right.
Thinking about the main parts of a problem helps programmers get a full picture of what they’re tackling. This knowledge lets them plan and make solutions that really tackle the problem’s core.
“Effective problem representation is the key to solving complex challenges in programming and beyond.” – John Doe, Software Engineer
Brute Force Approach
Programmers often use the brute force approach to solve problems. This method uses a computer’s speed to try every possible solution until it finds the right one. It’s good for simple problems but not always the best choice.
This algorithm checks every possible outcome without skipping any. It’s a sure way to find the right answer. But, it can make the program run slow and use a lot of resources.
Brute force algorithms come in two types: optimizing and satisficing. Optimizing algorithms look for the best solution. Satisficing algorithms aim for a good enough solution.
Pros of Brute Force Algorithms | Cons of Brute Force Algorithms |
---|---|
– Guaranteed method to find the correct solution – Versatility across problem domains – Simplicity in implementation – Serve as a baseline for comparisons | – Inefficiency for real-time problems – Exponential growth in algorithm analysis – Reliance on computational power – Slow execution – Lack of creativity compared to other design paradigms |
The brute force method is useful but not always the best choice. Programmers aim for better, smarter solutions. They use their knowledge of algorithms and computer science to improve their work.
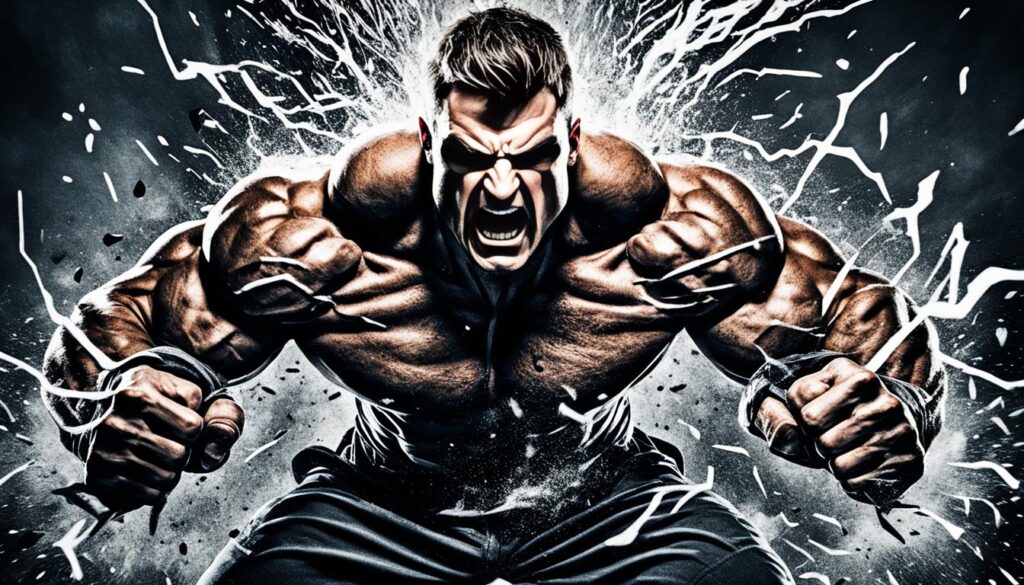
“The brute force approach is like trying to find a needle in a haystack by picking up every single piece of hay until you find it. It’s simple, but often not the most efficient solution.”
How Programmers Represent Problems or Systems
Programmers use many formal languages and notations to tackle problems or systems. This approach makes solving problems clear and standard. Tools like pseudocode, flowcharts, and Unified Modeling Language (UML) diagrams help in visualizing complex issues.
Formal Languages and Notations
Pseudocode gives a clear, step-by-step guide to an algorithm. It lets programmers plan the logic and flow of a solution without worrying about language rules. Flowcharts show the steps in a problem with symbols, making it simpler to grasp the control flow and decisions.
UML diagrams, such as class and sequence diagrams, are key tools. They model a system’s structure and behavior. This helps in sharing complex ideas and solving problems together.
Knowledge Representation Techniques
Programmers also use knowledge representation techniques to tackle complex problems abstractly. Logical inference and symbolic reasoning let them work with symbolic info. This helps in understanding problems deeply and creating stronger solutions.
By using formal languages, notations, and knowledge techniques, programmers can better capture, share, and think about problems. This leads to more efficient and successful problem-solving.
Practice and Problem-Solving
Improving your problem-solving skills takes time and regular practice. Programmers use “micro problem-solving” exercises like chess puzzles and coding challenges. These activities help them get better at breaking down and solving problems. This kind of practice is key to becoming a skilled problem-solver.
The 2018 Developer Skills Report by Hacker Rank shows that problem-solving skills are crucial for employers. They are more important than knowing programming languages or debugging. C. Jordan Ball, a top coder, says breaking down big problems into smaller ones is a great way to solve them. V. Anton Spraul believes new programmers should focus on problem-solving skills over just learning syntax.
Andrew Singer points out that debugging is vital in programming. It’s important to know what the program actually does, not just what you intended. C. Jordan Ball also suggests finding general principles to simplify problem-solving. The 2017 internet trends report by Mary Meeker shows that leaders like Elon Musk and Mark Zuckerberg got their problem-solving skills from playing video games.
“The obstacle is the way. Turn it into your advantage.” – Ryan Holiday
Improving problem-solving skills requires practice. You can do this with chess puzzles, math problems, coding challenges, or video games. These activities help improve your problem-solving abilities. Computational thinking is also useful. It means breaking problems down into smaller parts for better problem-solving.
Computational Thinking Characteristics | Description |
---|---|
Decomposition | Breaking down a complex problem into smaller, more manageable parts |
Abstraction | Focusing on the essential features and ignoring irrelevant details |
Pattern Recognition | Identifying common elements or trends in a problem |
Algorithm Design | Developing a step-by-step procedure to solve a problem |
By doing problem-solving practice regularly and using techniques like computational thinking, programmers can keep getting better at solving problems. This makes them more adaptable and effective at solving problems.
Conclusion
Programmers need to be great at solving problems. They must know how to break down issues, plan solutions, and fix errors. They also need to spot and use patterns to their advantage.
This skill set, along with ongoing learning and practice, helps programmers solve complex problems. It lets them make innovative software.
Understanding programming basics like computational thinking and problem-solving is key. These skills help programmers face many challenges confidently. They can adapt to new tech and make a mark in computer science.
As technology grows more important, the need for skilled programmers keeps rising. By improving their problem-solving skills and keeping up with new trends, programmers can become crucial in many fields. They’ll drive innovation and shape our digital future.
FAQ
How do programmers represent problems or systems?
Programmers use formal languages and notations to model complex problems. They use pseudocode, flowcharts, and UML diagrams. They also use logical inference and symbolic reasoning.
How do programmers explain problems in plain, simple language?
Programmers explain problems clearly, avoiding technical jargon. They focus on the main parts of the problem, like inputs, outputs, and what the system should do.
How do programmers plan the solution to a problem?
Programmers plan by outlining steps to solve the problem. They use comments in the code for clarity. This helps in understanding the problem-solving process.
How do programmers use a “divide and conquer” approach to problem-solving?
Programmers break big problems into smaller parts. They solve each part step by step. Then, they combine the solutions. This makes solving problems easier and helps with debugging.
How do programmers debug and troubleshoot their code?
When debugging, programmers use step-by-step methods to find errors. They’re open to reassessing their approach if needed. This mindset is key to solving problems.
How do programmers continuously learn and improve their problem-solving abilities?
Programmers keep learning by exploring new techniques and technologies. They practice with exercises like chess puzzles and coding challenges. This helps them solve problems better.
How do programmers represent and manipulate data?
Programmers use variables, data types, and structures to represent data. The way data is structured affects the solution’s efficiency and effectiveness.
What is the role of algorithms in programming?
Algorithms are key in programming. They provide step-by-step instructions for solving problems. Programmers use computational thinking to develop efficient solutions.
How do programmers use encapsulation and abstraction to manage complexity?
Programmers use encapsulation and abstraction to organize data and hide details. This makes large software easier to build and maintain.
How do programmers recognize and generalize patterns in problems?
Identifying patterns in problems is crucial for programmers. By spotting common themes, they can find or create reusable solutions. This helps them apply solutions to more problems.
How do programmers represent problems or systems in general terms?
Programmers focus on the main parts of problems, like variables and inputs. Variables change, while constants don’t. Clearly defining inputs and outputs is key to solving problems.
What is the “brute force” approach to problem-solving, and how do programmers avoid it?
The brute force method tries every possible solution quickly. It works for simple problems but not always. Programmers aim for more efficient, thoughtful solutions.
How do programmers use formal languages and notations to represent problems or systems?
Programmers use formal languages and notations like pseudocode and flowcharts. They also use logical inference and symbolic reasoning to model complex problems abstractly.
How do programmers practice and improve their problem-solving skills?
Programmers improve by practicing regularly. They solve puzzles, play games, and tackle coding challenges. This practice is key to becoming a skilled problem-solver.