JavaScript is a widely used programming language, but many developers dislike it. This article explores the reasons behind this dislike. It covers everything from its history to its quirks and challenges.
One major issue is its loosely typed nature. This means it doesn’t strictly check the types of data you use. Another problem is the confusion between “==” and “===” for comparing values. Then there are immediate invoked function expressions (IIFEs) and the trouble of mixing different data types.
These issues make JavaScript hard to work with, especially when different browsers have their own ways of handling it. This has led many developers to look for other options, like Blazor, for their web projects.
This article aims to explain why JavaScript is often seen as a source of frustration. It highlights the language’s limitations and what makes it hard for developers to work with.
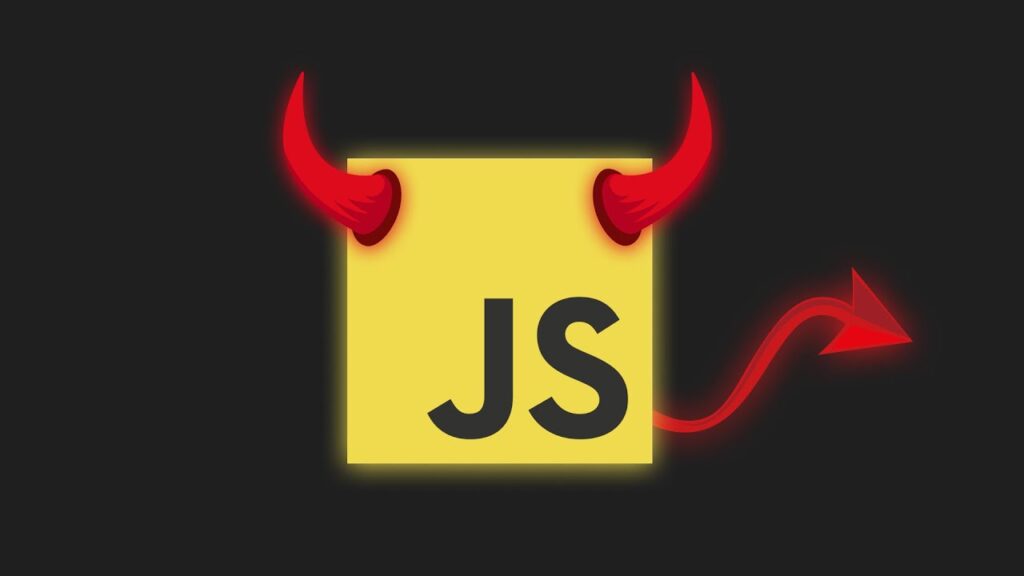
Key Takeaways
- JavaScript’s loosely typed nature and lack of defined data types can lead to unexpected behaviors and runtime errors.
- The confusion around the use of “==” and “===” comparison operators affects the outcome of equality checks in JavaScript.
- Immediate Invoked Function Expressions (IIFEs) in JavaScript can be a source of complexity and confusion for developers.
- Adding variables of different data types in JavaScript can result in unexpected outcomes due to the language’s type coercion behavior.
- JavaScript’s performance varies across different browsers, attributed to the use of different ECMAScript engines by various browser vendors.
JavaScript’s Turbulent History
JavaScript is a key language that powers the web today. It started in the mid-1990s, growing with the internet and web developers’ needs.
Brendan Eich’s 10-Day Challenge
In April 1995, Brendan Eich, a Netscape engineer, was tasked with creating a new language in just 10 days. Eich, known for his creativity, succeeded in making a language that changed web development.
Netscape’s Need for a Web Scripting Language
Netscape wanted a language for the web that worked with Java in the browser. This led to the creation of JavaScript, originally called Mocha and then LiveScript, but later named JavaScript. The name change was mostly a coincidence.
The 1990s saw the internet grow fast, making JavaScript more popular. Developers loved it for adding cool features to websites.
“JavaScript was created in 10 days in May 1995 by Brendan Eich, then working at Netscape, and officially launched as LiveScript in Netscape Navigator 2.0 in September of that year.”
JavaScript’s early days had ups and downs. It was loved but also criticized for its oddities. Yet, its strong community and creativity helped it grow and stay important in web development.
The Rise of JavaScript’s Popularity
JavaScript started with criticism but has become the most used programming language for web development. Its demand for jobs has soared, especially from 2019 to 2020. This surge in javascript popularity has made it a key player in web development, despite its challenges.
Now, over 16.4 million developers worldwide use JavaScript, making it the top language. Last year, two million more developers started using it, showing its fast growth.
There are many javascript job opportunities because of its role in making web pages and apps. A 2021 survey showed JavaScript is the top language, beating HTML/CSS and Python in use.
“JavaScript, invented by Brendan Eich in 1995 in just ten days, quickly became known as the ‘programming language of the web,’ emphasizing its rapid development and adoption.”
JavaScript has faced challenges, like the “Browser Wars” with Microsoft’s JScript. But it has stayed popular, with both fans and critics noting its unique qualities.
JavaScript’s future looks bright, with new areas like cryptocurrency and peer-to-peer networks drawing Brendan Eich’s interest. As web development grows, the need for skilled JavaScript developers will keep it vital in tech.
Reason 1: Loosely Typed Language
Programmers often dislike JavaScript because it’s loosely typed. This means variables can hold any type of value without declaring it first. This flexibility can cause unexpected issues, like a variable changing its type during runtime. For example, a variable might start as a number but then be changed to a string, leading to confusion and bugs.
JavaScript also has a unique concept called NaN (Not a Number). This is considered a number in JavaScript, which can confuse developers who prefer strict type checking in other languages.
Variables Without Defined Data Types
JavaScript lets variables hold any type of value, which can be both good and bad. It makes coding faster, but it can also cause unexpected problems and bugs. Developers need to pay close attention to how they manage variable types to keep their code stable and predictable.
NaN: A Number That Isn’t a Number
NaN (Not a Number) often confuses developers. When JavaScript does a calculation that doesn’t result in a number, it gets a NaN value, which is still seen as a number. This can lead to tricky errors that are hard to fix, especially for newcomers to the language.
“JavaScript’s dynamically typed nature can be both a blessing and a curse. The flexibility of not having to declare variable types can speed up development, but it can also lead to unexpected behavior and bugs.”
JavaScript’s loose typing and NaN can be tough for programmers, especially those used to strict type checking. Developers must be careful with variable types and understand JavaScript’s quirks to avoid problems and keep their code stable and predictable.
Reason 2: Double Equals vs. Triple Equals
The double equals (“==”) and triple equals (“===”) operators in JavaScript often cause confusion. The double equals operator changes the types of the operands before comparing them. This is known as type coercion.
The triple equals operator checks if the value and type are the same. This can lead to surprises. Double equals might say two values are equal even if they are different types. Triple equals will say they are not equal.
For example, consider these comparisons:
- “1” == 1 // true (type coercion)
- “1” === 1 // false (strict comparison)
- NaN === NaN // false (NaN is never equal to NaN)
Comparing NaN (Not-a-Number) with itself using triple equals always returns false. This is because JavaScript checks if one operand is NaN first, before comparing types.
To avoid type coercion issues, use the triple equals operator (“===”) for all comparisons in JavaScript. This is best unless you need to convert types. It makes your code clearer and more predictable.
“Understanding the implications of coercion and the differences between double and triple equals is crucial for writing clear and precise JavaScript code.”
Knowing how double and triple equals work helps you write better JavaScript. Your code will be more reliable and predictable, even with different data types.
Reason 3: Immediately Invoked Function Expressions (IIFEs)
In the world of JavaScript, Immediately Invoked Function Expressions (IIFEs) can be tricky. They let you define and run functions in one step. This helps keep variables private and stops them from messing with the global space.
The way to write an IIFE might seem odd at first. You wrap the function in parentheses, then add more parentheses to call it right away. This method is powerful but can be hard to grasp for beginners.
- IIFEs are functions that start right after they’re defined, making private variables and keeping the global space clean.
- The syntax, with the function in and then invoked with parentheses, can confuse those new to JavaScript.
- Even though IIFEs are useful, their purpose and how to use them can be unclear, causing frustration and the need for more learning.
javascript IIFEs and javascript self-executing functions are key in the JavaScript world. Learning about them is important for becoming a skilled JavaScript developer. But, they can be tough to understand at first.
“IIFEs are a powerful tool, but their syntax and purpose may not be immediately intuitive for developers new to JavaScript.”
The importance of IIFEs might change as JavaScript evolves, with new module syntax and features coming up. But knowing about IIFEs is still vital for JavaScript developers. It helps them understand the language’s core and design patterns.
Reason 4: Adding Different Data Types
In JavaScript, adding variables with different data types can be tricky. This often leads to unexpected results, especially for those used to other programming languages.
String Concatenation vs. Number Addition
When you mix a string and a number in JavaScript, it changes the number to a string. Then, it does string concatenation instead of adding numbers. This can surprise developers who expect a different outcome.
For instance, look at this code:
let x = 5;
let y = "10";
let result = x + y;
console.log(result); // Output: "510"
Here, the number 5 turns into a string and combines with “10”. This gives us “510”, not the expected 15.
This issue can be a big problem with user input or mixed data types. Developers must know this to make sure their code works right.
To add numbers correctly, you can change variables to numbers with parseFloat()
or Number()
. For example:
let x = 5;
let y = "10";
let result = x + Number(y);
console.log(result); // Output: 15
Knowing how to handle this is key for writing dependable JavaScript code.
Reason 5: Browser Inconsistencies
Programmers often find JavaScript frustrating because it doesn’t work the same way in all web browsers. This is because each browser has its own version of the ECMAScript standard. This standard defines how JavaScript should work.
For example, Google Chrome’s V8 and Mozilla Firefox’s SpiderMonkey interpret JavaScript differently. This means the same code can behave differently in each browser. It’s a big problem for developers who want their apps to work everywhere.
ECMAScript Engine Variations
Different browsers use different ECMAScript engines. This leads to various issues, such as:
- Varying levels of support for newer JavaScript features and syntax
- Inconsistent handling of edge cases and unexpected inputs
- Differences in optimization and performance characteristics
- Discrepancies in error handling and reporting
To fix these javascript browser inconsistencies, developers spend a lot of time testing and debugging. They also have to add special code to make sure their apps work on different browsers. This extra work is time-consuming and can be frustrating, especially for complex web projects.
“The lack of consistency in how javascript ECMAScript engines interpret and execute JavaScript code is a significant pain point for many developers.”
As the web changes, with new browsers and technologies, this problem will likely keep happening. Developers must stay alert and flexible when writing JavaScript that works across all browsers.
why programmers hate javascript
JavaScript is a key language for web apps, but many programmers dislike it. They find it hard to work with because of several issues. These problems make JavaScript a tough language to use.
One big reason is its loosely typed nature. JavaScript doesn’t clearly define what type of data a variable can hold. This can lead to unexpected results, like “100” being equal to true. While this makes quick prototyping easy, it also causes frustration for developers.
Another issue is the inconsistent behavior of JavaScript across different browsers. Each browser has its own way of handling JavaScript, making it hard for developers. They have to deal with many browser-specific problems.
“JavaScript was created in a matter of weeks with a sloppy implementation in the ’90s due to time constraints, and the perception of it being a poor language choice was influenced by the need to outmaneuver Sun’s Java strategically.”
JavaScript also has unique quirks and syntax. Things like Immediately Invoked Function Expressions (IIFEs) and the tricky “this” keyword make it hard for new developers to learn. This has made the learning curve steep.
- JavaScript was once seen as a “quick and dirty” language, and this view still exists. Many programmers dislike it for its early flaws.
- The fast pace of new tools and libraries in JavaScript has also caused frustration. Developers find it hard to keep up with changes.
- There are worries about JavaScript’s performance limitations compared to languages like Java, Golang, Rust, and Swift. There are also high costs in maintaining JavaScript apps.
Despite the criticism, the JavaScript community has grown and improved. ECMAScript 6 (ES6) and later versions have fixed some issues. Front-end frameworks and Node.js for server-side work have also helped developers. Yet, many still see JavaScript as a flawed and frustrating language. The debate about its value and limitations continues among programmers worldwide.
Embracing JavaScript’s Ecosystem
Many developers find JavaScript complex, but it has created a vast ecosystem that changed web development. This ecosystem includes powerful front-end frameworks and server-side JavaScript with Node.js. It’s a key player in web development today.
Front-end Frameworks and Libraries
JavaScript’s popularity led to many front-end frameworks and libraries. These tools help solve specific problems and make development easier. Examples are React, Angular, and Vue.js.
These frameworks offer structured ways to build web pages. They help with things like managing states and updating parts of the page efficiently. This makes building complex web interfaces easier for developers.
Back-end with Node.js
Node.js lets JavaScript run on servers, expanding its use. Developers can now create back-end applications with the same language they use for front-end work. This has led to many tools and frameworks for Node.js.
Tools like Express.js and Koa make building APIs and managing servers easier. The mix of front-end frameworks and JavaScript for back-end has made JavaScript a full solution for web apps. Despite its challenges, the ecosystem around it is crucial for developers who can work with its quirks.
Front-end Frameworks | Back-end with Node.js |
---|---|
React Angular Vue.js | Express.js Koa Nest.js |
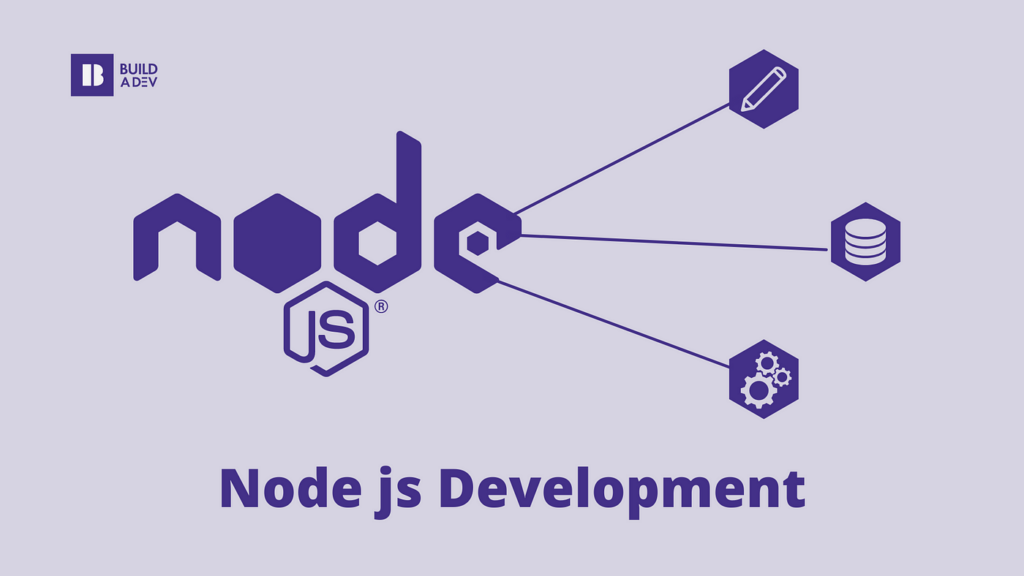
“The JavaScript ecosystem has become a comprehensive and versatile solution for modern web applications.”
The Frustrations of Dynamic Typing
JavaScript’s dynamic typing brings flexibility but also brings big frustrations for developers. It can change types without warning, leading to unexpected behavior. For example, adding a string and a number might give you a string instead of a number. This makes it hard to understand what your code will do, leading to tricky errors.
Type Coercion and Unexpected Behavior
JavaScript’s dynamic typing has a big problem with type coercion. This means it can change data types on its own. For instance, adding 1 and ‘2’ together gives you ’12’, not 3. This can be really frustrating for developers because it makes bugs hard to find and fix.
Another issue is how JavaScript handles NaN, or “Not a Number.” This happens when you try to do math with something that’s not a number, like this:
console.log(1 * 'hello'); // Output: NaN
This might seem simple, but NaN can cause big problems when it spreads through your code. It leads to results you don’t expect and more frustration for developers.
Operation | Expected Behavior | Actual Behavior |
---|---|---|
1 + ‘2’ | 3 | ’12’ |
1 – ‘2’ | -1 | -1 |
1 * ‘hello’ | Error | NaN |
The table shows how JavaScript’s type coercion can lead to unexpected behavior. This can make coding hard and introduce bugs. It’s a trade-off for flexibility, making it tough for developers to work with JavaScript.
“JavaScript’s dynamic typing can be a double-edged sword, offering flexibility but also introducing frustrating and unexpected behavior that can make code harder to reason about and debug.”
Variable Scoping and the ‘this’ Keyword
JavaScript’s way of handling variables and the “this” keyword can be tricky for many developers. The “var” keyword makes variables available in the whole function, even in nested blocks. This can cause issues and make the code hard to understand.
The “this” keyword also adds to the confusion. It changes based on how a function is called, not just its definition. This means “this” might point to an object you didn’t expect, making the code harder to understand.
Function-Scoped Variables and Closures
In JavaScript, “var” declares variables that can be used anywhere in the function. This can sometimes cause problems, as variables might be accessed outside their original scope. To fix this, “let” and “const” were added in ES6. They make variables only available in their block, making things clearer.
Closures add more complexity to variable scoping. They let inner functions use variables from the outer function, even after the outer function ends. This is powerful but can be tricky if not handled right.
The Confusing ‘this’ Context
The “this” keyword is another challenge for developers. Its value changes based on how a function is called, not just its definition. This can lead to “this” pointing to an object you didn’t expect, making the code hard to understand.
In ES5 strict mode, “this” is usually undefined, which can be helpful. But in non-strict mode or with IIFEs, “this” might point to the global window object. This adds to the confusion.
To control “this”, JavaScript has methods like “call”, “apply”, and “bind”. These let developers set “this” in a function. Knowing how “this” works and these methods is key to writing clear and predictable JavaScript code.
Keyword | Scoping | Redeclaration |
---|---|---|
var | Function-scoped | Allowed |
let | Block-scoped | Not allowed |
const | Block-scoped | Not allowed |
The table shows how var, let, and const work in JavaScript. It highlights their differences in scoping and how they handle redeclaring variables.
“JavaScript developers often dislike the dynamic nature of the ‘this’ keyword, leading some to avoid using it and opt for a more functional programming approach.”
Callback Hell and Asynchronous Nightmares
Developers often struggle with JavaScript’s asynchronous nature, leading to “callback hell.” This happens when many callbacks are used to manage tasks, making code hard to read and maintain.
JavaScript’s way of handling tasks can be tough for developers. They deal with callbacks, promises, and async/await. Managing these can make coding hard and lead to “callback hell.”
To fix this, the JavaScript world has come up with solutions like the Promise system in ES6 and async/await. These help make handling tasks easier and make code clearer.
But, JavaScript’s async nature still causes problems for many. Developers must think about the order of tasks, handle errors, and make sure results are passed correctly. This can make code complex and prone to errors, especially in big projects.
“Dealing with JavaScript’s asynchronous programming model is like navigating a minefield – one wrong step, and your code can blow up in your face.”
Despite the challenges, JavaScript’s widespread use has led to finding ways to overcome javascript callback hell and javascript asynchronous programming. Using tools like Promise chaining, async/await, and libraries for error handling can make coding easier.
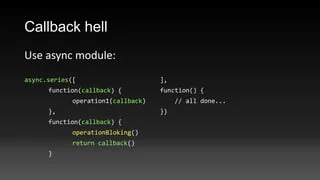
The JavaScript world is always changing. We can expect to see more improvements in how it handles tasks. This will make it easier for developers to write code that is easy to maintain, scalable, and efficient.
Blazor: A Beacon of Hope
Blazor has brought hope to developers tired of JavaScript. It’s a .NET framework that lets developers build web UIs with C# instead of JavaScript. This solves many problems developers face with JavaScript.
Component-Based Architecture
Blazor changed the game with its component-based architecture. It lets developers break down web interfaces into easy-to-manage parts. This makes coding easier, keeps things organized, and makes big projects simpler to handle.
Seamless Code Sharing
Blazor stands out for its easy code sharing between the server and client. Developers can write C# code that works everywhere, cutting down on duplicate work. This makes sure the server and client sides work together smoothly.
Blazor’s strong typing and error checking help fix common JavaScript problems. It offers a solid framework and uses C# power. This makes Blazor a great choice for developers looking to beat JavaScript challenges.
“Blazor is a game-changer for web development, offering a component-based architecture and seamless code sharing that address the pain points of working with JavaScript.”
Feature | Blazor | JavaScript |
---|---|---|
Language | C# | JavaScript |
Type System | Strongly Typed | Loosely Typed |
Code Sharing | Seamless between Server and Client | Typically Separate between Server and Client |
Development Experience | Familiar to .NET Developers | Can be Challenging for Developers New to JavaScript |
The Advantages of C#
JavaScript’s dynamic typing and runtime errors have long been a challenge for programmers. Moving to C#, a statically typed language, has greatly improved the development process. C#’s strong typing and compile-time error checking help spot issues early, cutting down on debugging time and boosting productivity. This immediate feedback and type safety are major benefits C# has over JavaScript.
Strong Typing and Compile-Time Checking
C# is a compiled language with a strong type system, offering advantages over JavaScript’s dynamic nature. Variables in C# have set data types, and the compiler checks for type errors at compile-time, not runtime. This stops many common problems, like trying to do math with the wrong data types, that trouble JavaScript developers.
Also, C#’s strong typing supports tools like IntelliSense and type-checking in IDEs like Visual Studio. Developers get to enjoy smooth code completion, refactoring, and debugging tools. These tools make the development process smoother and cut down the time spent on fixing runtime errors.
Feature | C# | JavaScript |
---|---|---|
Type System | Statically Typed | Dynamically Typed |
Compile-Time Checking | Strong | Weak |
Memory Management | Automatic Garbage Collection | Manual or Library-Based |
Performance | Faster Execution | Slower Execution |
C#’s strong typing and compile-time checking catch and fix errors early in development. This leads to more reliable and easy-to-maintain web applications.
Conclusion
JavaScript is still key for web development, but its issues have made developers look for other options. Blazor has become a strong choice with its unique design and the benefits of using C#. It offers a way to avoid JavaScript’s problems.
Using Blazor and .NET frameworks can make developers more productive and improve their work. It also makes web app development more enjoyable. JavaScript is still important, with frameworks like React, Angular, and Vue.js leading the way. But Blazor and C# are showing promise for a better web development path.
The decision between JavaScript and alternatives like Blazor depends on what each team needs. Yet, Blazor’s growing popularity and benefits hint at a new future in web development. It could be a fresh start away from JavaScript’s hurdles.
FAQ
What is the turbulent history of JavaScript?
Brendan Eich created JavaScript in April 1995 during a 10-day challenge. It was first named Mocha, then LiveScript, and finally JavaScript. The name was chosen despite its lack of relation to Java. Netscape needed a scripting language for the web that worked with Java, leading to JavaScript’s creation.
Why has JavaScript’s popularity risen so rapidly?
JavaScript has grown in popularity, becoming the top language for web development. The demand for JavaScript skills has soared, showing its importance. This growth has made JavaScript a key player in web development, despite its challenges.
Why do programmers dislike JavaScript’s loosely typed nature?
Programmers dislike JavaScript for its loose typing. It lets variables change data types without declaring them. This can cause unexpected issues, like the NaN (Not a Number) problem, which is hard for some to understand.
What is the confusion surrounding the use of “==” and “===”?
The double equals (“==”) and triple equals (“===”) operators in JavaScript confuse many. Double equals changes types to compare values, while triple equals checks both value and type. This can lead to wrong results, making developers frustrated.
What are Immediately Invoked Function Expressions (IIFEs) and why are they a source of confusion?
IIFEs are functions that start running right after they’re defined. They help keep variables private and avoid polluting the global space. But, their complex syntax can confuse new developers, leading to frustration and the need for more learning.
Why is adding variables with different data types in JavaScript challenging?
Adding variables of different types in JavaScript can be tricky. It turns numbers into strings for adding, not doing math. This can surprise developers who expect different results, especially those from other languages.
What are the challenges of JavaScript’s inconsistent behavior across different browser environments?
JavaScript works differently in each browser due to unique ECMAScript engines. This means code can behave differently in Google Chrome versus Mozilla Firefox. Developers face the challenge of making their code work everywhere, needing to test and adjust often.
What are the key frustrations that have led many programmers to dislike JavaScript?
Many dislike JavaScript for its loose typing and confusing syntax. Its behavior varies by browser, adding to the frustration. These issues make JavaScript a tough language to work with, despite its wide use.
How has the emergence of Blazor provided an alternative to JavaScript for web development?
Blazor has offered hope for developers tired of JavaScript. It uses .NET for building web UIs with C#, not JavaScript. Blazor’s strong typing and compile-time checks help solve many JavaScript problems, making development easier.
What are the key advantages of using C# over JavaScript for web development?
Moving to C# for web development has improved the experience for many. C#’s strong typing and error checking catch problems early, making development faster and more reliable. This is a big plus over JavaScript’s dynamic typing and runtime errors.