In the world of computer science, data structures are key to organizing and managing information. They help programmers make their code better, faster, and more efficient. By using the right data structures, developers can solve complex problems and create software that works well.
Data structures are vital in computer science. They affect how software works, uses memory, and processes information. Programmers need to think about what data they’re working with and how it will be used. They also need to consider memory and storage needs.
By picking the right data structure, developers can make their code run smoothly. This leads to efficient algorithms that handle tough tasks well.
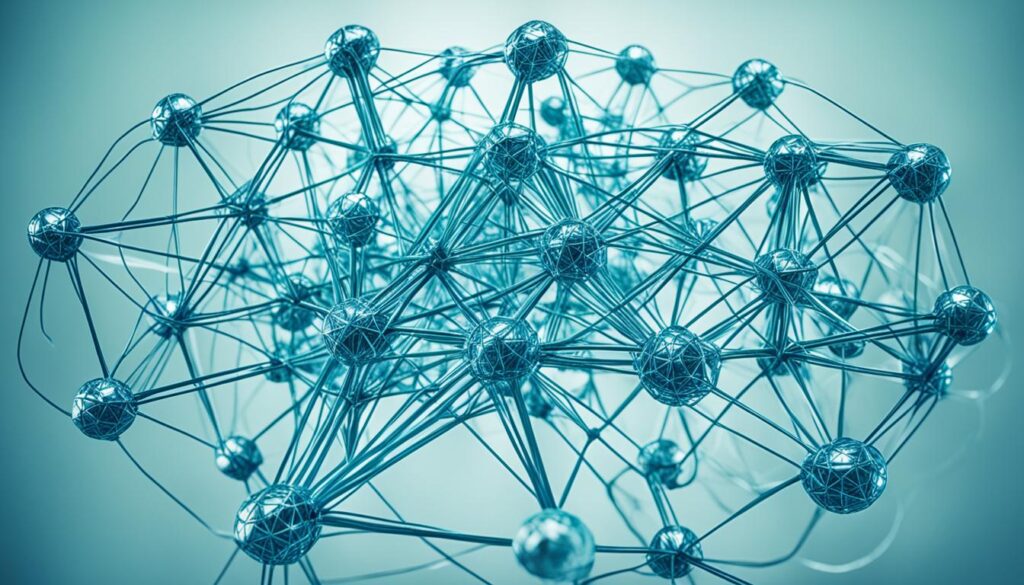
Key Takeaways
- Data structures are specialized formats for organizing, processing, retrieving, and storing data, crucial in computer science and programming.
- Programmers use data structures to improve algorithm efficiency, enhance code readability and maintainability, and optimize memory utilization.
- Choosing the right data structure for a task can help solve complex real-world problems more effectively and make software more scalable and performant.
- Data structures are foundational for organizing, managing, and processing data in computing tasks, influencing efficiency, memory usage, and processing time.
- Proper selection of data structures enables problem-solving in network analysis, task scheduling, and data modeling.
What Are Data Structures?
Data structures are special ways to arrange data for a certain goal. They give a clear model for how data is set up. This makes it easier to use, keep, and share information. Data structures are key for complex apps, as they help with algorithms and programs.
Defining Data Structures
Data structures tell us how to store, get to, and change data in a computer program. They decide how info is put together and handled. This choice can greatly affect how a program runs, uses memory, and works well.
Importance of Data Structures
- Data structures are key for handling and processing big amounts of data efficiently.
- They help programmers make more advanced and growing applications by offering a structured way to manage data.
- Knowing data structures is vital for understanding and using algorithms, which are key in computer programming.
- Being good at data structures shows a programmer can think deeply and solve hard problems, which is very valuable in tech.
- Understanding data structures makes developers’ programs run faster and use resources better.
In short, data structures are the basic parts of computer programming. They let programmers work with data well. Being good at data structures is a key skill for any software engineer or developer. It’s important for making strong and growing applications.
Efficiency in Data Organization and Manipulation
Data structures are key to organizing and handling data well. By picking the right data structure, programmers can make algorithms better and save resources like memory and time. This is very important in big projects that deal with a lot of data, as it can make things run much faster.
Choosing the right data structure is a big part of making algorithms and software. Each data structure is good at different things, and the choice depends on what the application needs. For example, arrays work well for storing a set number of similar items. Linked lists are better for adding or removing items easily.
The data structure you pick affects how fast and efficiently an algorithm works. Efficient data organization can make a big difference, especially in big projects with lots of data. Programmers need to know the strengths and weaknesses of different data structures to make smart choices and improve their code’s efficiency.
Arrays, linked lists, stacks, queues, trees, and graphs are all used in different situations. For example, B-trees and hash tables are important in databases for quick searching and sorting. New technologies like AI use data structures for learning and recognizing patterns.
As software development gets more advanced, the need for efficient data handling will keep growing. Future software engineers will need to know about both traditional and new data structures and their uses. This will help them create solutions that work well and use resources wisely.
“Efficient data organization can lead to significant performance improvements, especially in large-scale applications that handle massive amounts of data.”
Optimal Algorithm Performance
Data structures are key to making algorithms work their best. The choice of data structure affects how fast and efficient an algorithm is. By picking the right data structure, programmers can make their algorithms use less resources and run faster.
Algorithmic Complexity
Algorithmic complexity is a big deal in computer science. It shows how efficient an algorithm is. It’s measured using Big-O notation, which tells us the maximum time and space an algorithm needs as the input grows. Knowing about algorithmic complexity helps programmers pick the best data structures for their algorithms. This ensures their algorithms can handle big datasets without slowing down.
Examples of Algorithm Optimization
Here are some ways to improve algorithm performance with the right data structures:
- Using a binary search tree for quicker searches. These trees are faster than linear search, taking O(log n) time for searches, insertions, and deletions.
- Choosing a hash table for super-fast data access. Hash tables are great for quick lookups, insertions, and deletions, taking an average time of O(1).
- Using sorting algorithms like quicksort or mergesort for big datasets. These algorithms sort data faster than bubble sort or insertion sort, taking O(n log n) time.
- Opting for the B-tree for fast data access in large datasets. B-trees are great for big data, balancing storage and quick lookups.
Understanding the pros and cons of different data structures and their complexities helps programmers make smart choices. This leads to more efficient and resource-saving algorithms.
“Algorithmic techniques such as dynamic programming allow teams to optimize performance, streamline workflows, and solve complex problems confidently.”
Operation | Time Complexity | Space Complexity |
---|---|---|
Insertion (array) | O(N+1) | O(1) |
Deletion (array) | O(N) | O(1) |
Linear Search (array) | O(N) | O(1) |
Binary Search (sorted array) | O(log N) | O(1) |
Removal by value (unordered array) | O(N) | O(1) |
Removal by value (ordered array) | O(N) | O(1) |
Memory Utilization and Management
Designing data structures well is key to using memory efficiently in computer programming. The type of data structure used can greatly affect how much memory an application needs. This, in turn, impacts its performance and how well it can grow.
Arrays store data in a block, making it quick to access and store. Linked lists, however, let you add or remove data easily. Trees are great for organizing data in a way that saves memory.
When designing data structures, programmers must think about the data’s size, how it will be accessed, and how memory is allocated. Choosing the right data structure can save a lot of memory. This is very important when working with limited resources.
Hash tables are great for fast data lookups and use little memory. Dynamic arrays can grow or shrink based on the data, saving memory. This flexibility is useful when data needs change.
Caching and compression help manage memory too. By keeping often-used data in cache and compressing data, programmers can use memory more wisely. This makes applications run faster.
Data Structure | Memory Utilization | Strengths |
---|---|---|
Arrays | Contiguous memory allocation | Efficient storage and retrieval |
Linked Lists | Flexible memory allocation | Easy insertion and deletion |
Trees | Hierarchical memory organization | Efficient for structured data storage |
Hash Tables | Minimal memory overhead | Constant-time retrieval |
Dynamic Arrays | Adjustable memory allocation | Adaptive to changing data requirements |
Knowing how different data structures use memory and using smart memory management helps programmers make their applications better. This leads to faster performance and better use of resources, making for a smoother user experience.
Enhancing Code Readability and Maintainability
Designing data structures well is key to making software code easier to read and maintain. By organizing data in logical ways, programmers can write code that is clear and easy to work with. This makes it simpler to understand, fix, and change the code later.
Data structures help by putting data and operations together into abstract data types (ADTs). This lets programmers focus on what the code does, not how it does it. This makes the code clearer and easier to work on together, as everyone can see the big picture.
Also, good data structures make it easier to use the same code in different projects. By having standard data structures, programmers can share parts of their code. This saves time and keeps the development process consistent.
Benefit | Description |
---|---|
Improved Readability | Data structures provide a structured way to represent and organize data, making the code more intuitive and easier to understand. |
Enhanced Maintainability | Modular code with well-defined data structures is simpler to debug, modify, and extend over time, reducing long-term maintenance costs. |
Increased Reusability | Standardized data structures can be reused across different projects, promoting consistency and accelerating development. |
By focusing on designing and using effective data structures, programmers can make code that is efficient, easy to read, and easy to keep up with. This leads to better software and a more productive way of working.
why programmers use data structures ?
Data structures are key in computer programming. They help programmers organize and manage information well. Programmers use them to make algorithms faster, use memory better, and solve complex problems.
Programmers use data structures to make their algorithms work better. The right data structure can save time and storage. For instance, a binary search algorithm can find what you need in just 30-40 seconds.
Data structures are also important for managing memory. Different data types need different amounts of memory. Choosing the right data structure helps use memory wisely, making software run better.
- Enhancing code readability and maintainability: Well-structured data makes code easier to understand and work with.
- Solving complex real-world problems: Data structures help organize information in ways that make processing easier. This lets programmers solve big problems, like managing social networks or finding words in a dictionary.
Knowing about data structures and algorithms is key for job interviews in the tech world. Programmers who know these skills are sought after by top companies. They show they can create efficient and scalable solutions.
In summary, programmers use data structures to make their code better and solve big problems. The right data structure saves time and makes code easier to read and update. Learning about data structures and algorithms is essential for success in today’s tech world.
Applications in Searching and Sorting Techniques
Data structures are key in using searching and sorting algorithms. They help sort and search through data efficiently. Arrays, linked lists, and trees are used for different types of searches and sorts. These tools work together to make software run better and faster.
Searching Algorithms
Searching means finding something in a collection of data. Programmers use linear and binary search often.
- Linear search looks at each item one by one until it finds what you’re looking for. It’s simple but can take a long time for big datasets.
- Binary search is faster and works on sorted data. It cuts the search area in half at each step. This makes it much quicker than linear search.
Sorting Algorithms
Sorting puts data in order, like from smallest to largest. There are many sorting algorithms, each with its own benefits. Some top ones are:
- Insertion sort moves items around to keep them in order. It inserts new items in the right spot.
- Merge sort breaks the data into smaller parts, sorts them, and then puts them back together.
- Quicksort picks a pivot and sorts the rest of the data around it.
- Heapsort uses a heap to sort the data efficiently.
Choosing the right algorithms depends on the data size and type, and what the application needs. Picking the right ones can make software run much better.
Using data structures and the right search and sort algorithms is crucial for making software efficient. By knowing how these work, programmers can solve complex problems and meet user needs.
Common Data Structures Used by Programmers
Programmers use various data structures to make their code better and faster. These include arrays, linked lists, stacks, and queues. Each one has its own use and benefits for different tasks.
Arrays
Arrays store items in memory close together. This makes getting to elements fast. They’re often used in many algorithms and apps. Arrays help create other data structures like array lists and vectors.
Linked Lists
Linked lists are like arrays but use pointers to connect elements. They’re great for adding or removing items easily. They’re important in compiler design and come in different types for different needs.
Stacks and Queues
Stacks and queues keep elements in order, either LIFO or FIFO. Stacks are used for math expressions and recursive calls. Queues help manage threads and are used in queuing systems, including priority ones.
Data Structure | Description | Key Applications |
---|---|---|
Arrays | Contiguous memory storage of items, allowing efficient indexing and retrieval | Implementing other data structures, sorting algorithms, database indexing |
Linked Lists | Dynamic, pointer-based structure for efficient insertion and deletion | Symbol table management in compilers, circular linked lists for task switching |
Stacks | Last-in-first-out (LIFO) order for expression evaluation and function calls | Parsing and evaluating mathematical expressions, implementing recursion |
Queues | First-in-first-out (FIFO) order for managing threads and queuing systems | Multithreading, priority queues, and other queuing applications |
Programmers use data structures like arrays, linked lists, and stacks to work with data. These tools help make apps run better and do more.
Trees and Their Applications
Trees are a key data structure that store data in a hierarchical way. They are used in many areas, like file systems and search engines. This structure helps programmers make algorithms better for searching, sorting, and getting data.
There are different types of trees, each with its own benefits. For example, binary search trees are great for fast searches and updates. Space partitioning trees like K-D Trees are vital in computer graphics and machine learning.
B-Trees and B+ Trees are often used in databases for indexing. Suffix trees are excellent for finding patterns in text quickly. This makes them useful for certain text-based tasks.
Tries are used to make dictionaries with quick lookups. Decision trees are key in machine learning for solving problems and predicting outcomes.
In networking, spanning trees and shortest path trees help with data transmission. XML parsers also use tree structures to handle XML data efficiently.
Tree traversal methods like in-order and pre-order help in accessing data in trees. In graphics and UI design, trees help organize data for better visuals and user experience.
Trees are great for balancing speed and flexibility in programming and data management. By knowing how different trees work, programmers can solve complex problems more efficiently and elegantly.
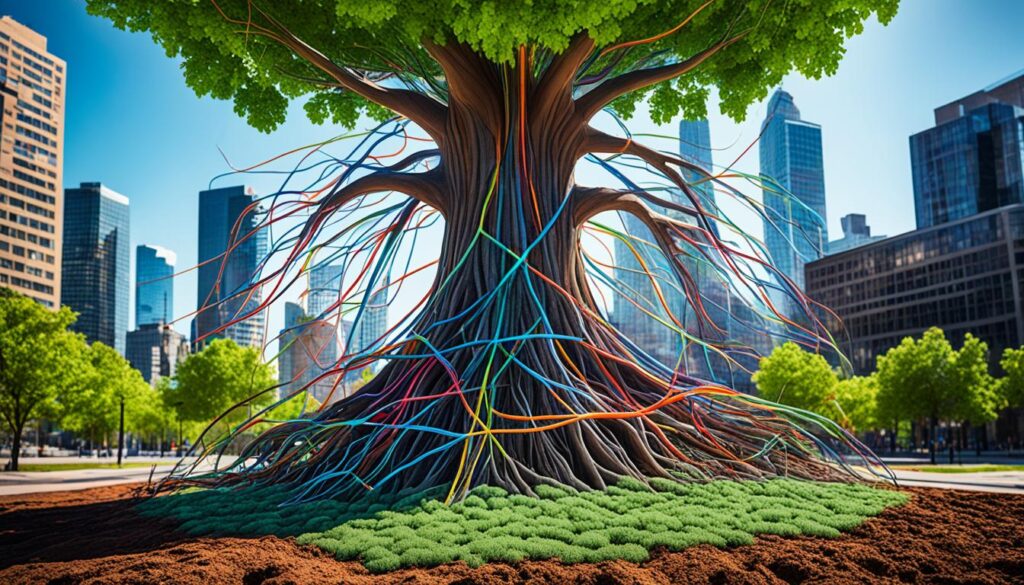
“Trees are the lungs of our land, purifying the air and giving fresh strength to our people.”
– Julius Sterling Morton
Graphs and Their Real-World Uses
Graphs are a key tool for programmers to understand complex systems. They are made up of nodes and edges that connect them. This lets programmers work with the relationships between objects easily.
Graphs are used in many areas, like social media, transportation, and computer networking. They help programmers understand and improve these systems. This makes them very useful.
In social media, graphs show how users connect. This helps find key people or groups. For transportation, graphs help plan routes and find the best paths.
Application | Relevance of Graphs |
---|---|
Social Media | Represent user connections and analyze social networks |
Transportation | Model road networks and optimize routing algorithms |
Computer Networking | Depict network topologies and optimize data flow |
Network Analysis | Identify patterns, clusters, and influential nodes in complex systems |
Graphs are also used in family trees, scheduling, and citation networks. They help programmers solve complex problems by showing relationships. Tools like Minimum Spanning Trees (MSTs) and Directed Acyclic Graphs (DAGs) are key in these tasks.
In short, graphs are vital for programmers. They help solve complex problems by showing how objects relate. From social media to transportation, graphs are a key tool in modern software development.
Data Structures in Interviews and Hiring
Data structures and algorithms are key in technical interviews for software engineering jobs. They test a candidate’s problem-solving skills and their ability to pick the right data structures for complex problems. Interviewers give coding challenges that need specific data structures like arrays or graphs to see if a candidate can apply them in real situations.
Interview Question Examples
Candidates often face questions about data structures and algorithms in technical interviews. These questions check if a candidate can design efficient solutions and improve code performance. They also test problem-solving skills. Here are some common data structure-related interview questions:
- Implement a stack or queue using an array or linked list
- Traverse a binary tree in different orders (in-order, pre-order, post-order)
- Find the shortest path between two nodes in a graph using a breadth-first search (BFS) or depth-first search (DFS) algorithm
- Implement a hash table to store and retrieve key-value pairs efficiently
- Optimize the time complexity of a sorting algorithm (e.g., merge sort, quick sort)
- Solve a problem using a recursive or dynamic programming approach
These questions check a candidate’s knowledge of data structures and algorithms. They also look at their critical thinking, communication skills, and ability to write efficient code.
Candidates who do well in technical interviews know the basics of data structures and their complexities. They can apply these to solve different problems. They can also improve algorithms, making them stand out to companies looking for skilled software engineers.
By getting good at data structures and algorithms, candidates can show off their problem-solving skills. This can help them get software engineering jobs at top tech companies.
Solving Complex Real-World Problems
Data structures and algorithms are key in solving complex problems across many industries. They help programmers make innovative solutions. These tools make software more efficient and scalable. Let’s look at real-life examples that show how data structures and algorithms change things for the better.
Revolutionizing Transportation and Logistics
In transportation, graph data structures help with road networks and making routes better. They turn roads, intersections, and travel times into nodes and edges. This lets algorithms find the shortest paths, reduce traffic, and make deliveries faster.
This has improved traffic management, fleet use, and last-mile delivery. It helps businesses and customers alike.
Enhancing Social Media Experiences
Social media uses graph algorithms to connect users and suggest new friends. By seeing user relationships as a graph, these algorithms find key users, spot communities, and suggest connections. This makes social media better for users, increases engagement, and helps businesses understand their users.
Optimizing Financial Applications
In finance, data structures like hash tables and binary search trees are used to quickly find and manage financial data. These tools make it fast to look up, add, or remove data. This is key for handling lots of transactions, managing investments, and making reports.
By using the right data structures, programmers can solve complex problems and make new solutions. As technology grows, the need for skilled programmers will too. They must know how to use data structures and algorithms to tackle big challenges. This makes these concepts vital for making software.
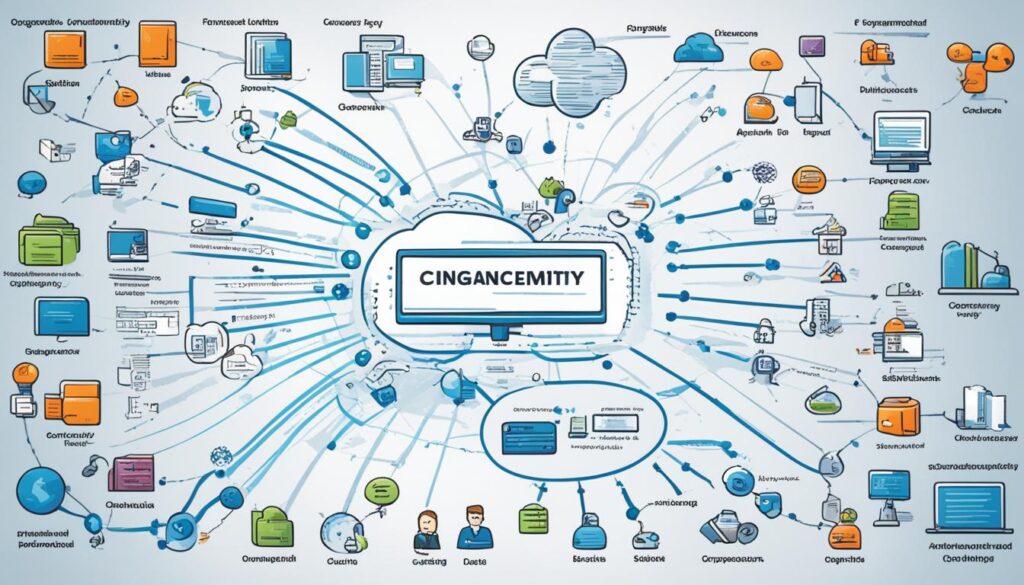
“The power of data structures and algorithms lies in their ability to transform complex problems into manageable and efficient solutions that can drive real-world progress.”
Choosing the Right Data Structure
Choosing the right data structure is key for programmers. It affects how well a program works, how much memory it uses, and how easy it is to read. When solving a problem, programmers must think about the data type, the operations needed, and the solution’s requirements.
When picking a data structure, algorithm performance is a big factor. Different structures have different times and space complexities for things like searching and inserting. Knowing the pros and cons of each structure helps programmers make their algorithms run better.
Memory usage is also crucial. The data structure chosen can greatly affect a program’s memory use. Programmers need to balance memory use and performance, considering the problem’s needs.
Code readability and maintainability matter too. Some structures, like linked lists or trees, make the problem easier to understand and change later.
To pick the best data structure, programmers should fully understand the problem. They should look at the data types, operations needed, and the problem’s requirements. This helps narrow down the best data structures for the job.
- Identify the key operations needed, like searching or inserting.
- Look at the time and space complexity of these operations for different structures.
- Think about memory use and how it affects the program’s performance.
- Consider how easy the code is to read and maintain with the chosen structure.
- Try out different structures and algorithms, comparing their performance on sample data.
By thinking carefully and experimenting, programmers can pick the best data structure for their needs.
“The choice of data structure can make the difference between a program that is merely correct and one that is efficient and effective.”
Data Structure | Strengths | Weaknesses |
---|---|---|
Arrays | Efficient for random access, simple implementation | Fixed size, expensive for insertion/deletion |
Linked Lists | Dynamic size, efficient for insertion/deletion | Slower random access, more memory overhead |
Trees | Efficient for hierarchical data, fast searching | Complexity in implementation and balancing |
Hash Tables | Constant-time access, efficient for searching | Sensitive to hash function, potential collisions |
Understanding the strengths and weaknesses of different data structures helps programmers make better choices. This leads to more efficient and effective software.
Conclusion
Data structures are key in computer science. They help organize and manage digital information in a structured way. By using the right data structures, programmers can make algorithms run better, make code easier to read and keep, and solve complex problems.
The choice of data structure greatly affects how efficient and scalable software is. They are vital for managing databases, network communications, and powering AI and machine learning. As data grows and gets more complex, using data structures wisely will be even more important for programmers and developers.
Learning about data structures and algorithms is a great skill for tech interviews and software engineering. It also opens doors to high-paying jobs at top tech companies. By learning and practicing these concepts, programmers can improve their problem-solving skills, write more efficient code, and set themselves up for success in the fast-changing tech world.
FAQ
What are data structures and why are they important?
Data structures help organize, process, and store data in a logical way. They are key in computer science and programming. They make information easier to work with. This improves how algorithms work, makes code easier to read and keep up, and uses memory better.
How do data structures impact algorithm performance?
The type of data structure used affects how fast and efficient an algorithm is. Choosing the right data structure can make algorithms use less resources and run faster. For example, using a binary search tree makes finding data quicker than a linear search.
How do data structures affect memory usage?
Using data structures wisely is important for managing memory well. There are static and dynamic data structures, each needing different amounts of memory. Programmers must think about data size, how it’s accessed, and memory strategies to use resources efficiently.
How do data structures improve code readability and maintainability?
Data structures make code easier to read and keep up by organizing information in a structured way. They put data and operations together into logical units. This makes code modular, easy to understand, and simpler to fix or change later.
What are some common data structures used by programmers?
Programmers often use arrays, linked lists, stacks, queues, trees, and graphs. Each has its own benefits and is chosen for the problem at hand.
How are data structures used in real-world problem-solving?
Data structures are vital in solving complex problems in many areas. For example, they help model transportation networks with graph data structures or efficiently store and find financial data with hash tables.
How do data structures and algorithms factor into the hiring process for software engineering roles?
In interviews for software engineering jobs, data structures and algorithms are key. They test a candidate’s problem-solving skills, algorithmic thinking, and their choice of data structures for complex problems.