In the world of software development, functions are key for programmers. They are blocks of code that do specific tasks. This makes writing code faster and cleaner. Programmers use Python’s built-in functions or create their own to save time and improve their work.
Functions boost reusability, making code easier to use again. They also make modularity and abstraction better.
This article will show why programmers use functions. We’ll look at the main reasons and coding rules behind it. By learning about functions, readers will see how they make software development better.
Key Takeaways
- Functions make code reusable, cutting down on repetition and making it easier to maintain.
- They help make programs modular, making it simpler to test and fix parts separately.
- Functions make code easier to read and keep up with, especially in big projects.
- They make testing and debugging easier by wrapping up certain actions.
- Functions help control how a program flows and organize its logic in a clear way.
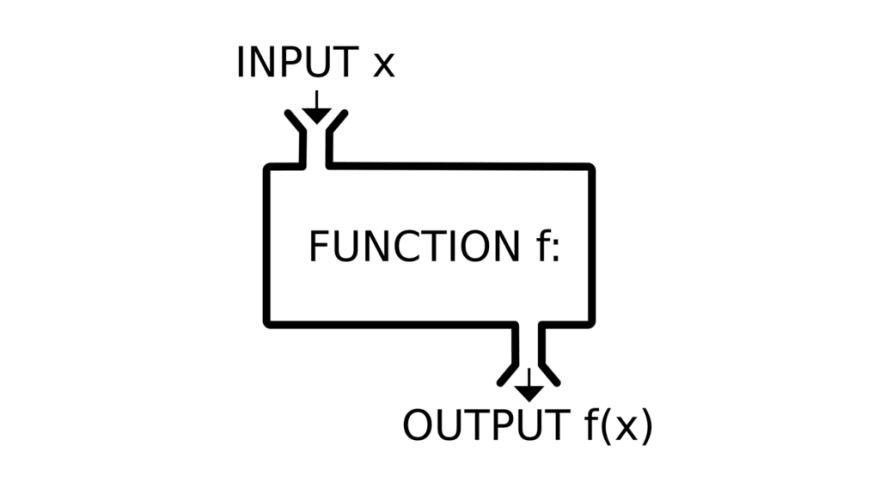
What are Functions?
Functions are key parts of programming languages. They are blocks of code that take in input, do specific tasks, and might give back a value. These blocks are important for making programs easy to manage and grow.
Definition and Purpose
A function in programming is a set of named steps that can be called to do a job. They help organize code, making it simpler to get, test, and use again. This way, complex problems can be split into smaller tasks, making code reusable and improving program structure.
Basic Example
Let’s look at a simple function that adds two numbers and gives the total:
“The function `add_numbers(a, b)` takes two numbers, `a` and `b`, adds them, and returns the sum.”
This example shows how functions take in input, do a task, and give back a value. Putting this into a function makes the code more organized, reusable, and easy to grasp.
Compound Statements and Code Blocks
Before we dive into functions, let’s get to know compound statements and code blocks. These are groups of statements that work together as one, often in curly braces. Code blocks help programmers define where variables are used and group instructions together. This is key for using functions.
Programming experts say to use indentation and compound statements to show code blocks, even if there’s just one statement. Different languages have their own ways to mark code blocks, like curly braces {}
in some or just using indentation in others.
In C++, it’s important for compilers to handle up to 256 levels of nesting for blocks. But, some tools like Visual Studio might not go that deep. It’s best to keep function nesting to 3 levels or less for easy reading.
Nesting Level | Recommendation |
---|---|
Up to 3 levels | Recommended for maintaining code readability |
More than 3 levels | Consider refactoring functions into sub-functions |
C++ programs can have many nested blocks, but it’s wise to keep it to 3 levels or less. If you’re using too many, think about breaking functions into smaller ones. This makes your code easier to understand and maintain.
“A code block, also known as a compound statement, is a fundamental lexical structure in programming languages that groups together one or more declarations and statements.”
Languages that let you create blocks, including nested ones, are called block-structured programming languages. Most if-then-else setups need specific indentation or syntax to mark code blocks.
Knowing about compound statements and code blocks helps programmers organize their code better. It helps them manage variables and set up a strong base for using functions. Functions are key in building software.
Anatomy of a Function
Functions are the core of programming. They let developers tackle complex issues by breaking them into smaller, reusable parts. Each function has key parts that shape its structure and how it works.
Function Name
The function name helps identify it in the code. It should clearly show what the function does. For example, a function to find a rectangle’s area could be named calculateRectangleArea.
Parameters and Arguments
Parameters are the inputs a function takes, shown as variables in the definition. Arguments are the actual values given when the function is used. For example, a rectangle area function might take length and width as parameters. When called, it could get 5 and 10 as arguments.
Return Type
The return type tells us what kind of data the function gives back. It can be a simple type like int or double, a custom data structure, or void if it doesn’t return anything. In our rectangle area example, the return type could be double since the area is a decimal number.
Knowing about function anatomy helps programmers design and use functions better. Functions are key to structured programming. They help break down big problems into smaller, manageable parts. This makes code more organized and efficient.
Calling Functions
In programming, functions are key to organizing code into reusable pieces. You call a function by its name, inside parentheses. The values in the parentheses are arguments, which match the function’s parameters.
When you call a function, the program runs the function’s code and then goes back to where it was called. This makes the code easier to read and manage. It also makes it reusable.
Here’s a simple example in C programming to show how to call functions:
#include <stdio.h> int add(int x, int y) { return x + y; } int main() { int result = add(5, 10); printf("5 + 10 = %d", result); return 0; }
In this example, the add()
function is called from the main()
function. It gets the values 5
and 10
as arguments. The function adds them and returns the result, which is then printed.
To call a function, you just need to say the function’s name and put its arguments in parentheses. This is the same in many programming languages like C, C++, Java, Python, C#, and JavaScript.
Functions are crucial for making code modular and reusable. They help break down big problems into smaller, easier parts. This makes creating and running programs simpler.
why programmers use functions ?
Have you ever wondered why functions are key in coding? They bring many benefits that make your code better, faster, and easier to keep up with. Let’s dive into why programmers use functions in their work.
Code Reusability
Functions are great for making code reusable. They wrap up a task or logic in one spot. This way, programmers can use that function over and over instead of writing the same code again. It saves time, cuts down on mistakes, and keeps the code consistent.
Abstraction
Functions also help with abstraction. They let programmers focus on the what and why of a task without getting into the details. This makes the code easier to read, keep up with, and understand.
Modularity
Breaking big programs into smaller functions makes them easier to handle. This approach helps organize the code better. It makes testing, fixing, and working together on projects simpler.
Readability and Maintainability
Functions are key to making code clear and easy to keep up with. They put specific tasks or logic into well-named blocks. This makes the code explain itself, helping others (or you later on) get and change the program easily.
In short, why programmers use functions is all about the benefits of functions. These include code reusability, abstraction, modularity, readability, and maintainability. These perks make functions a must-have for writing efficient, organized, and growing software.
“Functions are the building blocks of any programming language, enabling modularity, reusability, and abstraction – the cornerstones of effective software development.”
Code Reusability
Programmers use functions to make their code reusable. This means they can use the same code over and over without rewriting it. It saves time and helps avoid mistakes.
Using reusable code makes developers more productive and lowers costs. It also makes the software better. There are two ways to reuse code: using your own code or someone else’s.
Code reuse means developers can work faster and focus on new tasks. It also makes the code safer and smoother for users. But, it can be hard to share and keep up with reusable code.
Microservices architecture helps with code reusability. It lets developers use the same code in different parts of the system. Before reusing code, it must be checked for quality and reliability.
Tools like BrowserStack test code on many devices, making sure it works well. Reusable code is tested and reliable, saving time and reducing errors.
Breaking code into small parts makes it easier to use again in different projects. Object-oriented programming helps create code that can be reused easily. Good documentation and version control make it easier for others to use the code too.
Design patterns make creating reusable code simpler. Functions are key in coding. They let you store a task in a block and call it easily, avoiding the need to rewrite code.
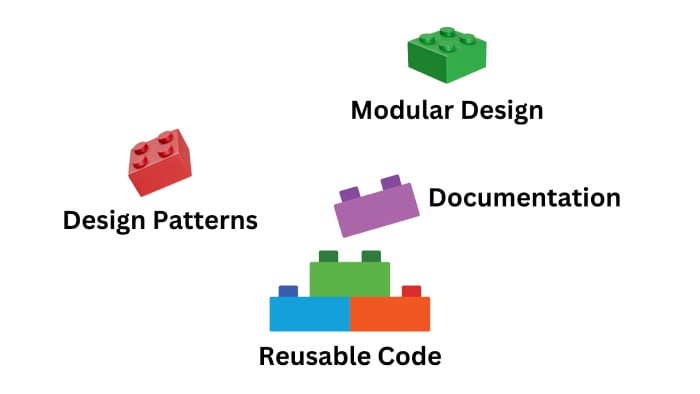
In JavaScript, functions are everywhere. They are used when you see parentheses, unless it’s in a loop or if statement. JavaScript has many built-in functions that add extra features and use languages like C++.
You can also create your own custom functions in JavaScript. These can be used for specific tasks. Functions can have different parts and even call other functions. They often need parameters to work right, which are values you add when you call the function.
Functions can have default parameters, which are values set in the function. This makes it easier to use the function without giving all the values. JavaScript also lets you create anonymous functions, which are useful in certain situations.
Function expressions define functions without a name. They are useful when you need to pass functions as arguments to other functions. Arrow functions are another way to write functions in JavaScript. They are great for simple tasks and need only one parameter.
Modularity
Modularity is key in software development. It lets programmers break down big tasks into smaller parts. By using a modular approach, developers make self-contained, reusable code pieces called functions. These functions are like building blocks, helping organize the program’s logic into separate modules.
Modular programming started in the 1960s to make software better organized and easier to maintain. Today, it’s a big part of how we make software, supported by languages like C, C++, Python, and Java.
At its heart, modular programming means breaking a big program into smaller parts. These parts, or modules, can be worked on, tested, and updated on their own. This makes the whole system less complex. By putting specific tasks in modules, programmers can easily get, change, and grow the program over time.
One big plus of modularity is code reusability. By making functions that can stand alone, developers can use them in different parts of the program or even in other projects. This saves time and cuts down on repeated code, making development more efficient and consistent.
Modularity also makes a program easier to maintain. When updates or fixes are needed, developers can work on one module at a time without messing with the whole system. This way, changes are safer and easier to manage.
In the case of the TinyMCE open-source WYSIWYG editor, modularity is key. The TinyMCE code is in a monorepo, one big repository with many modules for different tasks. This setup lets developers work on parts without affecting the whole system, making it more flexible and scalable.
By using modularity, programmers can make software that’s organized, easy to keep up with, and can grow. As software development keeps changing, the idea of modular design will stay important. It helps make complex programs better, faster, and more reliable.
“Modularity is a powerful concept in software engineering, allowing developers to create complex systems by assembling smaller, reusable components. This approach promotes code organization, reusability, and maintainability – key factors in delivering high-quality software solutions.”
Abstraction
In programming, functions are key to abstraction. They hide the complex details of a task, making it simpler for users or other parts of the program. This way, programmers can focus on what the function does without getting into its details.
Abstraction is vital in software engineering. It makes learning easier and work more efficient. Just like driving a car doesn’t mean knowing how the engine works, abstraction lets programmers use functions without diving deep into their details.
A great example of abstraction is the mean() function in Python. Programmers don’t need to know how it calculates averages. They just call the function and get the result, focusing on their main task.
Abstraction isn’t just for programming; it’s key in many areas like philosophy, art, mathematics, and electrical engineering. In electrical engineering, professionals work at various abstraction levels, from designing components to solving complex problems.
For example, in embedded systems, some engineers use low-level languages like C and assembly to work directly with hardware. Others focus on the high-level design and programming without needing to know the details.
Abstraction helps programmers work at a level closer to human thought. It makes code easier to organize and solve problems more efficiently. By hiding complex details, it lets developers focus on the problem’s essential aspects.
“Abstraction is the removal of unnecessary detail in engineering. Engineering cost and errors are reduced through abstraction.”
In summary, abstraction is crucial in programming. It helps manage complexity, improve code reusability, and maintainability. This leads to more efficient and robust software solutions.
Readability
In programming, making code easy to read is key. Functions with clear names make a big difference. They break down hard tasks into simpler parts. This makes the code easier for everyone to understand, even if they’re new to the project.
Good code readability comes from how we name functions and variables. Developers should use names that clearly show what the code does. Names like “create,” “set,” and “find” help make the code clear. It also helps if the function’s purpose is clear from its name.
Variables should have names that tell you what they hold. This makes the code easier to read. It also helps with understanding what a class instance does. A single sentence should explain what the instance is for.
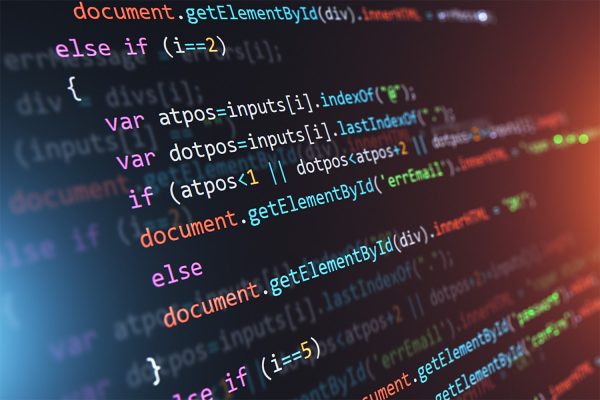
Code that’s easy to understand lets new developers get the hang of it quickly. It means they don’t have to dive deep into every detail. This makes code readability better and helps teams work together smoothly.
By focusing on function names and clear variable names, programmers make code that explains itself. This makes the software better and helps teams work faster and more together.
Metric | Impact on Code Readability |
---|---|
Cyclomatic Complexity | High cyclomatic complexity can slow down code reading and should be used judiciously, especially in high-performance scenarios. |
Static Imports | Static imports in Java should be used sparingly to avoid code ambiguity and ease of maintenance. |
Code Oddities | Documenting code oddities or instances where conventions are violated can greatly aid in code maintenance and understanding. |
By following these code readability tips, programmers can make software that’s efficient and easy to keep up with. This way, the software can grow and change as needed. It helps the project succeed over time.
Maintainability
Functions make it easier to update a program by letting you focus on specific parts. If you need to change something, you can just look at the function without checking the whole code. This saves time and effort in keeping the code up to date.
Handling a big codebase can feel overwhelming, but functions help a lot. They keep related code together, making it simpler to update certain parts without messing up the rest. This way, changes are easier to understand and fix.
A study from the University of Nebraska-Lincoln in 2023 showed how important code maintainability is. It found that the design of programming languages affects how easy it is to keep the code manageable. Readability, simplicity, and familiarity are key to making code easier to handle over time.
Functions fit well with these ideas of maintainability. They break down complex tasks into smaller, reusable pieces. This makes the code more organized and easier to understand. It also makes it simpler to make changes without causing problems elsewhere in the software.
“Functional programming encourages the separation of concerns and the creation of small, composable functions, promoting code reusability and modularity.”
In summary, using functions in programming is key to keeping software projects working well over time. They make it easier to make changes and keep the code organized. This helps developers update and improve the codebase more easily.
Testing and Debugging
Functions are key in testing and debugging software for developers. They break down big programs into smaller parts. This makes it easier to test each part separately. It helps find and fix problems faster, making the software better.
Debugging is finding and fixing errors in code. It’s a big part of making software. To debug well, you need to reproduce the bug, find the bad code, and fix it. Then, test the fix and document everything.
There are many ways and tools to help with debugging. Techniques like brute force and backtracking help find problems. Tools like IDEs and debuggers make developers work better and improve software quality.
Debugging Approach | Description |
---|---|
Brute Force | Testing all possible inputs to find the issue. |
Backtracking | Looking at how the program ran to find the problem. |
Cause Elimination | Getting rid of possible causes to find the real problem. |
Static Analysis | Looking at the code without running it. |
Dynamic Analysis | Watching how the program runs to find issues. |
Debugging is vital because it fixes bugs in software. There are many types of errors, like syntax and runtime errors. These errors can make the software not work right.
Using functions helps make code easier to test and debug. This leads to better software quality. It makes developers work more efficiently and gives users a better experience.
Control Flow
Functions are key in organizing the control flow of a program. They help developers make the program’s flow clear and easy to follow. This makes the program easier to understand and keep up with.
The control flow is how the program runs its code in order. This order is set by function calls, which take the program to a certain function and then back. Knowing about control flow is vital for writing clear and structured programs.
Conditional statements, like if
, else if
, and else
, are often used to control the flow. They let developers decide what to do next based on certain conditions. About 80% of developers use these statements to guide their programs.
Loops are also key in over 90% of programs. They help developers go through data or do tasks over and over. This makes the program’s logic and tasks more efficient.
Also, exception handling tools like try
, catch
, and finally
are used in more than 85% of projects. These tools help developers handle unexpected problems and keep the program running smoothly.
By carefully placing function calls, developers can make their programs more structured and easy to read. This method helps break down complex problems into simpler parts. It makes the program easier to understand and fix if needed.
In summary, knowing about control flow and how functions work is crucial for writing good code. Using techniques like conditional statements, loops, and exception handling makes programs better organized, easy to read, and strong.
Encapsulation
Functions in programming languages are key to encapsulation. This means hiding how a system works from the rest of the program. By using clear input and output, programmers keep the inner workings safe. This makes the code stronger and safer, as only the function’s public parts are seen by others.
Encapsulation is big in object-oriented programming (OOP) but less talked about in functional programming (FP). In OOP, it means hiding how an object works while still letting it do its job. It’s about making sure other parts of the program don’t mess with the object’s inner workings.
An example is a restaurant table object. It has rules for reserving seats. In languages like C#, classes follow these rules to keep things consistent. In functional programming, data doesn’t change and rules are kept through new values.
Functional programmers might not say “encapsulation” much, but they still focus on making data safe and correct. They use “smart constructors” to create data types that follow certain rules. They care more about how functions and data behave than traditional OOP ideas.
Encapsulation makes code easier to read, keep up, and make strong. It hides how things work, making code more modular and less likely to cause problems. This idea is key in making software secure and dependable.
“Encapsulation is the mechanism of hiding the internal implementation details of an application from the outside world.” – Anonymous
Function Design Principles
When designing functions, it’s key to follow certain principles for their effectiveness and upkeep. Two main principles to remember are the Single Responsibility Principle and Meaningful Function Names.
Single Responsibility Principle
The Single Responsibility Principle means a function should only change for one reason. It focuses on a single task. This makes functions more modular, easier to grasp, and less likely to have bugs. Functions with many tasks are tough to test, keep up, and reuse.
To follow this principle, keep these tips in mind:
- Make sure each function does a specific task.
- Don’t mix different tasks in one function.
- If a function is getting too big or complex, split it into smaller ones.
- Check your functions often to spot and fix any Single Responsibility Principle issues.
Meaningful Function Names
Choosing meaningful and descriptive function names makes the code easier to read and understand. These names give a clear idea of what the function does. This helps other developers (or you later on) get the code faster.
Here are some tips for naming functions:
- Use verbs or verb phrases that describe what the function does (like
calculateTotalCost()
orsendNotification()
). - Avoid names like
doSomething()
orprocess()
that are too vague. - Stick to a naming convention in your code, like camelCase or snake_case.
- Think about the function’s place in your app or library and use relevant terms in the name.
- Names should be short but clear enough to show what the function does.
By using the Single Responsibility Principle and meaningful function names, you make your functions better. They become more modular, easier to understand, and fit your code better. These ideas are part of the function design principles that help programmers write better code.
Conclusion
Functions are key in programming, offering many benefits to developers. They help make code organized, easy to keep up with, and run better. Using functions brings code reusability, makes things more modular, and makes the code easier to read and keep up with.
Functions in C programming make code reusable and cut down on repetition. This makes the code easier to handle. They also make complex things simpler, making the program easier to grasp. There are different kinds of functions, like library and user-defined ones, which let developers add their own custom code.
C programming functions come in different types, based on what they take in and give back. This lets developers plan their code better. By following best practices, like the Single Responsibility Principle, and using clear names, programmers can make functions that are easy to read and maintain. This way, they can test and fix functions on their own, making sure their apps work well.
FAQ
What is the purpose of using functions in programming?
Programmers use functions to make their code better, more reusable, and easier to handle complex tasks. Functions help organize code, make it simpler, and easier to keep up with changes.
What is a function in programming?
A function is a block of code that can be used again and again. It takes in input, does specific tasks, and might return a value. Functions are key in programming, letting developers break their code into smaller, easier parts.
What are compound statements and code blocks?
Compound statements group several statements together as one unit, often in curly braces. These blocks help define where variables are used and group instructions together. They’re a step towards using functions.
What are the key components of a function?
A function has a name, parameters, and a return type. The name identifies it, parameters are the inputs, and the return type is the data type of the output.
How do you call a function?
You call a function by its name, with arguments in parentheses. These arguments match the function’s parameters.
Why do programmers use functions?
Programmers use functions for many reasons. They make code reusable, modular, and easier to read and maintain. They also help with testing, control flow, and keeping code organized.
How do functions promote code reusability?
Functions let developers reuse code by grouping related tasks together. This saves time and reduces bugs. It makes the code more efficient and reliable.
How do functions contribute to modularity?
Functions break down complex tasks into smaller parts. This makes the code easier to understand and maintain. It helps developers work on different parts of the program separately.
How do functions enable abstraction?
Functions hide complex details, showing only what’s needed. This makes the code simpler and easier to use. Developers can focus on what the function does without getting into the details.
How do functions improve code readability and maintainability?
Good function names make code easier to read and understand. Breaking tasks into functions makes the code clear and straightforward, even for new people.
How do functions contribute to the testing and debugging process?
Functions make testing easier by letting developers check specific parts of the code. This helps find and fix problems faster, making the software better.
How do functions influence the control flow of a program?
Functions help organize how the program runs. By placing function calls carefully, developers can make the flow clear and easy to follow. This makes the program easier to understand and keep up with.
How do functions contribute to the principle of encapsulation?
Functions help keep code safe by hiding how it works. They define clear inputs and outputs, protecting the internal details from outside changes or access. This makes the code stronger and more secure.
What are the key principles for designing effective functions?
Good function design follows the Single Responsibility Principle and uses clear, descriptive names. This makes the code easier to read and understand.