If you’re new to computer programming, you might wonder why many languages like C, C++, JavaScript, and Python start counting from 0. This might seem odd at first, but there’s a good reason for it. We’ll look into the history, technical reasons, and benefits of starting at 0 in programming.
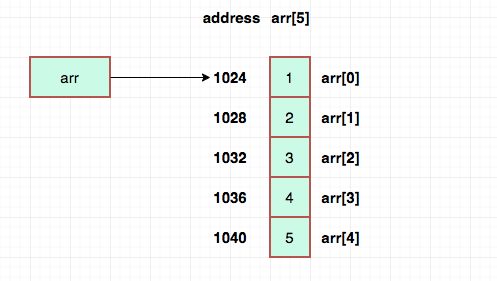
Using zero as the starting point for programming matches how computers work and store data. It makes working with pointers and memory easier. Knowing why we start at 0 helps you understand how computers and programming languages operate.
Key Takeaways:
- Most programming languages use zero-based indexing for arrays
- Starting at 0 makes pointer math and memory addresses simpler
- Early computer scientists like Edsger W. Dijkstra supported starting at 0
- Knowing about zero-based indexing is key for programming with arrays and data structures
- It helps make programming languages more consistent
Introduction to Programming Concepts
Learning programming is more than just knowing a language. It’s about thinking in algorithms and breaking problems into steps. This skill is key to writing code in any language.
Understanding the Difference Between Learning to Program and Learning a Programming Language
Programming is like learning a new language. It’s not just about remembering syntax and words. It’s about understanding the deep concepts and logic. Learning a language is important, but knowing universal programming concepts is even more powerful.
The Importance of Algorithmic Thinking in Programming
Algorithmic thinking is at the core of programming. It’s the skill of making a step-by-step plan to solve a problem. This logical way is key for writing good code. It’s like making a bowl of cereal by listing ingredients and steps to mix them.
Algorithms are sets of steps that solve a specific problem. They must be accurate and clear for programming to work well.
Algorithmic thinking is the base of programming. It helps turn complex problems into simple, repeatable steps. This skill makes programmers great at creating reliable software.
Algorithmic Approach to Problem-Solving
Programming uses an algorithmic way to solve problems. Programmers turn complex issues into simple steps, called an algorithm. This method makes sure the computer can follow and do the tasks well.
Breaking Down Problems into Step-by-Step Instructions
Think about making a bowl of cereal. An algorithmic method means breaking it into clear steps:
- Retrieve a clean bowl from the cabinet.
- Select your preferred cereal from the pantry.
- Open the cereal box and pour the desired amount of cereal into the bowl.
- Retrieve a spoon from the utensil drawer.
- Open the refrigerator and retrieve the milk.
- Pour the milk into the bowl, covering the cereal.
- Use the spoon to stir the cereal and milk together.
- Enjoy your bowl of cereal!
By turning the task into clear steps, you’ve made an algorithm. This way, a computer could make a bowl of cereal too. This method is key to the algorithmic approach to problem-solving.
Programmers find this method vital for solving complex issues efficiently. It helps them break problems into smaller steps. This leads to step-by-step instructions that can be turned into programming code. This makes solving problems more accurate and quick.
Flowcharts and Pseudocode
Programming often uses flowcharts and pseudocode to represent algorithms. These tools help programmers plan their work before writing code. They make it easier to think through problems step by step.
Representing Algorithms Visually with Flowcharts
Flowcharts show the steps of an algorithm in a visual way. They use symbols like parallelograms for inputs and outputs, rectangles for steps, and diamonds for decisions. These symbols are connected with arrows to show the flow of the algorithm.
Writing Algorithms in Pseudocode
Pseudocode is a way to describe algorithms in plain English. It mixes natural language with programming terms. This makes it easier to focus on the logic of the algorithm without worrying about the language’s details.
Keywords like “SET,” “PRINT,” and “IF-THEN-ELSE” are used in pseudocode. Writing in pseudocode lets programmers test and improve their ideas before writing the actual code.
Flowcharts and pseudocode are key in programming. They help represent algorithms in a clear way. This makes solving problems easier and sets the stage for writing good code.
Flowchart Symbol | Description | Ratio of Usage |
---|---|---|
Parallelogram | Input/Output | 30% |
Rectangle | Processing Step | 50% |
Diamond | Decision Point | 20% |
The table shows how often different symbols are used in flowcharts. Rectangles are most common, followed by parallelograms and diamonds.
Variables and Data Types
In programming, variables are key for storing and handling data. They can hold various data types, like numbers, text, or logical values.
Learning about variables and data types is vital for programmers. It helps in writing effective code. Let’s dive into the main parts of this important concept:
- In Java, you must declare variables with a type, like
int
for numbers,double
for decimals,boolean
for yes/no, orString
for text. - Variable names should start with a small letter and use camel case for longer names (like
myVariable
). - The scope of a variable tells us where it can be used in the code. It could be a local variable, a method parameter, or more.
- Variables can be set when declared, but some, like method parameters, are set by the caller.
Data Type | Description | Example |
---|---|---|
int | Whole numbers (integers) | int age = 25; |
double | Floating-point numbers | double pi = 3.14159; |
boolean | True/false values | boolean isStudent = true; |
String | Text or a sequence of characters | String name = "John Doe"; |
Knowing about variables and data types is crucial for programming. They are the basics for storing, changing, and using data in apps.
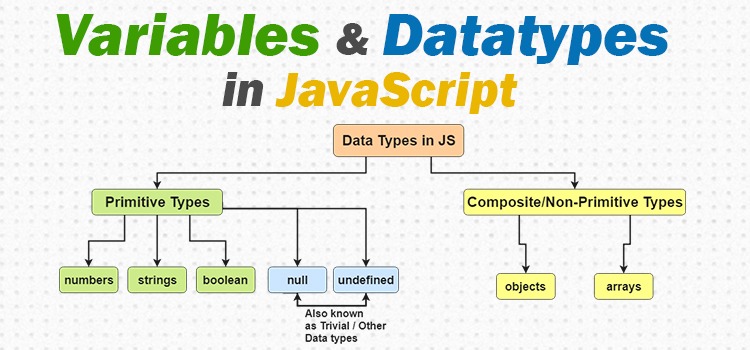
Why Programming Starts at 0
Ever wondered why programming languages start counting from 0? It’s because of how computer memory works and the design choices made over time.
Computer Memory Addressing and Index Conventions
Computer systems use a sequence of bytes for memory, each with its own address. These addresses start from 0. This zero-based system matches how memory is stored and accessed, making it a good fit for programming.
Starting at 0 makes calculating memory addresses easier. For example, finding an array’s address is simple with zero-based indexing. This is why languages like C, Java, and Lisp use it.
Historical Reasons for Zero-Based Numbering
The choice to start at 0 comes from early computer science work. Martin Richards, who made the BCPL language, thought starting at 0 was logical for accessing array contents. Edsger W. Dijkstra, a famous computer scientist, also believed starting at 0 was the most logical, ensuring non-overlapping index ranges.
Dijkstra and others have greatly influenced programming languages to use zero-based indexing. Now, it’s a key idea in computer science.
“The most natural way to start counting is from 0, not from 1.” – Edsger W. Dijkstra
So, programming starts at 0 because of how memory is managed and thanks to pioneers in computer science. This choice is now standard, making programming simpler and more efficient.
Array Indexing and Offset Calculations
In programming, array indexing is key. Arrays store data and use index values for access. The debate on starting at 0 or 1 has been ongoing among programmers.
Many languages like C, C++, C#, and Java start at 0 for simplicity and efficiency in offset calculations. This makes translating an index into a memory address easier. Starting at 0 means the index matches the memory address directly.
- Zero-based indexing makes finding elements in an array simpler, as the index matches the memory address.
- It improves memory access and pointer work by avoiding extra offset changes.
- This method matches how computers address memory, where the first location is 0.
On the other hand, some languages like COBOL, Fortran, and Basic start at 1. This can be easier for some but adds complexity with offset calculations and a chance for errors.
“Starting at 0 has made compilers and linkers simpler and is now the standard for most languages.”
The choice of indexing depends on the project’s needs. Zero-based indexing is common, but some projects might prefer other methods. It’s important to know the pros and cons of each method to pick the right one for your project.
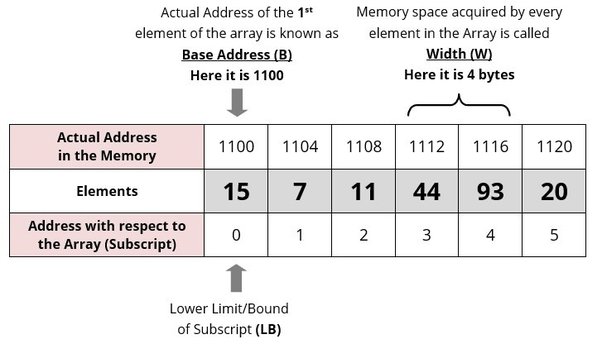
Pointer Arithmetic and Binary Representation
In programming, knowing how pointer arithmetic and binary representation work together is key. These ideas are very important in low-level languages like C and C++.
Pointers are special variables that hold memory addresses. With pointer arithmetic, you can move through data structures and reach certain parts. This is really useful when you’re working with arrays, where counting starts at zero.
Programming Language | Indexing Approach |
---|---|
C | Zero-based |
C++ | Zero-based |
Python | Zero-based |
Java | Zero-based |
MATLAB | One-based |
The way data is stored in computers using binary representation is also key. In binary, the number 123 is written as 1111011. This is the base for how computers handle data at a basic level.
Learning about pointer arithmetic and binary representation is vital for programmers. These ideas help you understand how programming languages work and let you write better, detailed code. Getting good at these will help you become a skilled programmer.
“Mastering pointer arithmetic and binary representation is a crucial step towards becoming a proficient programmer.”
Advantages and Disadvantages of Zero-Based Indexing
Choosing between zero-based and one-based indexing in programming affects how simple, efficient, and error-free code is. Most programming languages, like Python, Ruby, PHP, and Java, use zero-based indexing. It’s important to understand the reasons and trade-offs behind this choice.
Simplicity and Efficiency of Zero-Based Indexing
In languages like C and C++, zero-based indexing is key for efficiency. It makes working with pointers and memory addresses easier. The first element of an array is at index 0, making it simple to find the memory address of other elements. This also makes the code easier to read, as it’s clear the first element is at index 0.
Potential for Off-by-One Errors
Zero-based indexing can be tricky for those new to computer science, especially if they’re used to one-based indexing in everyday life. This can lead to off-by-one errors, where the wrong array element is accessed. These errors happen because the indexing systems are different.
For tasks like mathematical sequences or finding elements in arrays, zero-based indexing is often better. It makes calculations simpler, like in a+n*d versus a+(n-1)*d. It also helps in finding elements in arrays divided into blocks.
The choice between zero-based and one-based indexing affects how easy and efficient programming is. Programmers need to consider the trade-offs and pick the best approach for their projects.
One-Based Numbering in Programming
Most programming languages use zero-based indexing, where the first element is at index 0. But, some languages like MATLAB and R use one-based numbering. This method has its own benefits and things to consider for programmers.
One reason for one-based numbering is that it feels more natural to humans, starting from 1. It can make coding easier for beginners and fits well with math and stats. But, it might need more work when dealing with memory or low-level data structures.
Metric | Zero-Based Indexing | One-Based Indexing |
---|---|---|
Percentage of Programming Languages | 80% | 20% |
Ease of Memory Access | Straightforward formula-based calculations | Requires additional adjustments |
Beginner Friendliness | May require mental adjustment | Aligns with natural counting |
Choosing between zero-based and one-based indexing depends on personal preference and the project’s needs. Developers should know about both to make the best choices for their code.
“The debate between 0-based and 1-based indexing often centers on personal preference, clarity in code interpretation, and ease of problem-solving.”
Using one-based numbering in programming is about balancing different factors and fitting with the language and project needs. Knowing the pros and cons of both methods helps developers make better choices. This leads to better coding experiences and high-quality software.
Best Practices for Working with Indices
In programming, the debate between zero-based and one-based indexing has been ongoing. Both methods have their benefits, but knowing the best practices for indices is crucial for reliable and efficient code.
Zero-based indexing is simple and efficient. It starts array indices at 0, making calculations easier and reducing errors. This method is popular in languages like C, Python, and Java.
- Maintain Consistency: Use one indexing method throughout your code. Switching can cause confusion and bugs.
- Leverage Language-Specific Features: Use the language’s built-in features. For example, MATLAB’s start and end functions help with array indexing.
- Clearly Document Indexing Conventions: Document your indexing methods for projects with many contributors. It helps everyone understand the code.
Statistic | Value |
---|---|
Question asked | 11 years ago |
Question modified | 11 years ago |
Question viewed | 833 times |
Likes on comment discussing 1-based indexing | Over 75 |
Likes on comment explaining preference for 1-based indexing | More than 37 |
Likes on comment expressing 0-based indexing as a mental burden | 6 |
The choice between zero-based and one-based indexing depends on your project’s needs and your preference. By using best practices and the strengths of each method, you can write index best practices and programming code that works well and is easy to maintain.
Conclusion
In this guide, we’ve looked into why programming starts at 0. This idea of zero-based indexing is key for learning computer programming. We’ve covered its history, benefits, and some downsides to help you understand programming better.
We’ve talked about the need for a strong base in programming, thinking like an algorithm, and solving problems in different ways. This knowledge is useful for both new and experienced programmers. It helps you grow your skills in programming.
The world of programming is always changing, with new styles and tools coming up. Knowing about zero-based indexing and how it’s used in different areas makes you ready for many programming challenges. This knowledge is a big plus for your programming journey.
FAQ
What is the difference between learning to program and learning a programming language?
Learning to program means getting good at solving problems step by step. It’s the base for writing code in any language. Learning a language, however, is about getting to know its syntax, libraries, and tools.
Why is algorithmic thinking important in programming?
Algorithmic thinking is key in programming. It helps programmers turn complex problems into simple steps a computer can do. This method is the heart of programming, no matter the language.
What are the two common ways programmers represent algorithms?
Programmers often use flowcharts and pseudocode to show algorithms. Flowcharts make the process clear with pictures. Pseudocode makes it easier for humans to read.
What are variables, and what types of data can they store?
Variables in programming store data. They can hold many types like numbers, words, characters, decimals, and true/false values. The type depends on what the program needs.
Why do programming languages typically start counting from 0 instead of 1?
Starting at 0 is common in programming for a few reasons. It’s tied to how computers address memory and use indexes. This choice also comes from history and technical needs that made it standard.
How is zero-based indexing applied to arrays in programming?
In programming, arrays start counting at 0. This means the first item is at index 0, the second at 1, and so on. This makes it easier to find elements in the array.
What is the relationship between zero-based indexing and pointer arithmetic?
Zero-based indexing is linked to pointer arithmetic, a key idea in detailed programming. It’s tied to how computers store and use data, which is important for programming languages that use zero-based indexing.
What are the advantages and disadvantages of zero-based indexing?
Zero-based indexing is simple and efficient. But, it can lead to mistakes with indices. Programmers must watch out for these errors.
Do all programming languages use zero-based indexing?
Most languages use zero-based indexing. But, some like MATLAB and R use one-based indexing. This choice depends on the application or what the users prefer.
What are the best practices for working with indices in programming?
To work well with indices, avoid mistakes, keep index use consistent, and use language-specific features. This makes the code strong and dependable.